<StackPanel xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:c="clr-namespace:WpfApplication1" Width="300" Height="300"
Name="Page1">
<StackPanel.Resources>
<c:MyData x:Key="myDataSource"/>
<c:MyConverter x:Key="MyConverterReference"/>
<Style TargetType="TextBlock">
<Setter Property="FontSize" Value="15"/>
<Setter Property="Margin" Value="3"/>
</Style>
</StackPanel.Resources>
<StackPanel.DataContext>
<Binding Source="{StaticResource myDataSource}"/>
</StackPanel.DataContext>
<TextBlock Text="Unconverted data:"/>
<TextBlock Text="{Binding Path=TheDate}"/>
<TextBlock Text="Converted data:"/>
<TextBlock Name="myconvertedtext" Foreground="{Binding Path=TheDate,Converter={StaticResource MyConverterReference}}">
<TextBlock.Text>
<Binding Path="TheDate" Converter="{StaticResource MyConverterReference}"/>
</TextBlock.Text>
</TextBlock>
</StackPanel>
//File:Window.xaml.cs
using System;
using System.Globalization;
using System.ComponentModel;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Data;
using System.Windows.Media;
namespace WpfApplication1
{
public class MyConverter : IValueConverter
{
public object Convert(object o, Type type,object parameter, CultureInfo culture){
DateTime date = (DateTime)o;
switch (type.Name)
{
case "String":
return date.ToString("F", culture);
case "Brush":
return Brushes.Red;
default:
return o;
}
}
public object ConvertBack(object o, Type type,object parameter, CultureInfo culture){
return null;
}
}
public class MyData: INotifyPropertyChanged{
private DateTime thedate;
public MyData(){
thedate = DateTime.Now;
}
public DateTime TheDate
{
get{return thedate;}
set{
thedate = value;
OnPropertyChanged("TheDate");
}
}
public event PropertyChangedEventHandler PropertyChanged;
private void OnPropertyChanged(String info)
{
if (PropertyChanged !=null)
{
PropertyChanged(this, new PropertyChangedEventArgs(info));
}
}
}
}
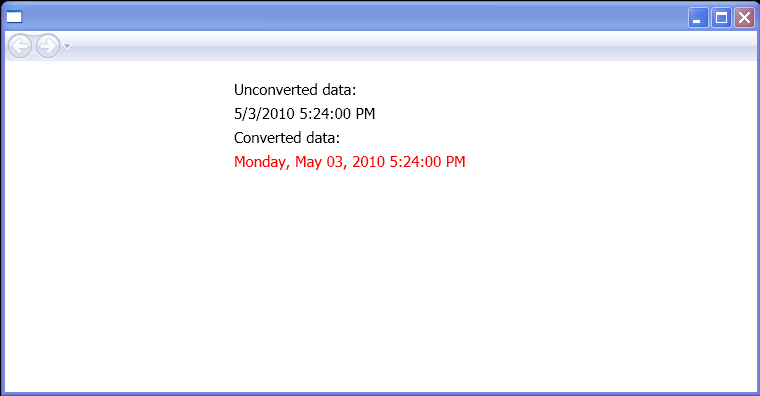