<Window x:Class="ClassicControls.CheckBoxList"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="CheckBoxList" Height="300" Width="300">
<Grid Margin="10">
<Grid.RowDefinitions>
<RowDefinition Height="*"></RowDefinition>
<RowDefinition Height="Auto"></RowDefinition>
</Grid.RowDefinitions>
<ListBox Name="lst" SelectionChanged="lst_SelectionChanged" CheckBox.Click="lst_SelectionChanged" >
<CheckBox Margin="3">Option 1</CheckBox>
<CheckBox Margin="3">Option 2</CheckBox>
<CheckBox Margin="3">Option 3</CheckBox>
</ListBox>
<StackPanel Grid.Row="1" Margin="0,10,0,0">
<Button Margin="0,10,0,0" Click="cmd_CheckAllItems">Examine All Items</Button>
</StackPanel>
</Grid>
</Window>
//File:Window.xaml.cs
using System;
using System.Collections.Generic;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
namespace ClassicControls
{
public partial class CheckBoxList : System.Windows.Window
{
public CheckBoxList()
{
InitializeComponent();
}
private void lst_SelectionChanged(object sender, RoutedEventArgs e)
{
if (e.OriginalSource is CheckBox)
{
lst.SelectedItem = e.OriginalSource;
}
if (lst.SelectedItem == null) return;
Console.WriteLine(lst.SelectedIndex);
Console.WriteLine(((CheckBox)lst.SelectedItem).IsChecked);
}
private void cmd_CheckAllItems(object sender, RoutedEventArgs e)
{
foreach (CheckBox item in lst.Items)
{
if (item.IsChecked == true)
{
Console.WriteLine((item.Content + " is checked."));
}
}
}
}
}
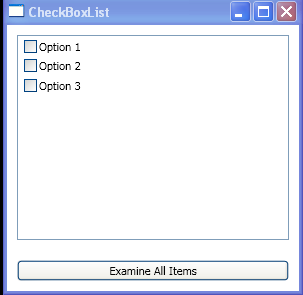