1. | String Object: =, Is and Mid | |  |
2. | String InStr | |  |
3. | String Insert | |  |
4. | Use the Mid function to replace within the string | |  |
5. | Use String.Equals methods | | 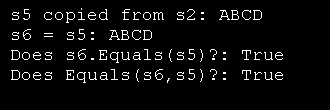 |
6. | Two ways to copy strings | | 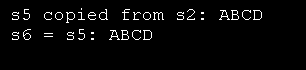 |
7. | Two ways to concatenate strings | |  |
8. | Sub string with only one parameter | | |
9. | String PadLeft | | 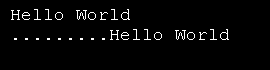 |
|
10. | String Constructor | | 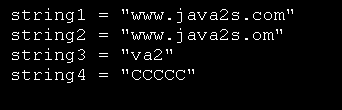 |
11. | String properties Length and Chars and method CopyTo of class String | | 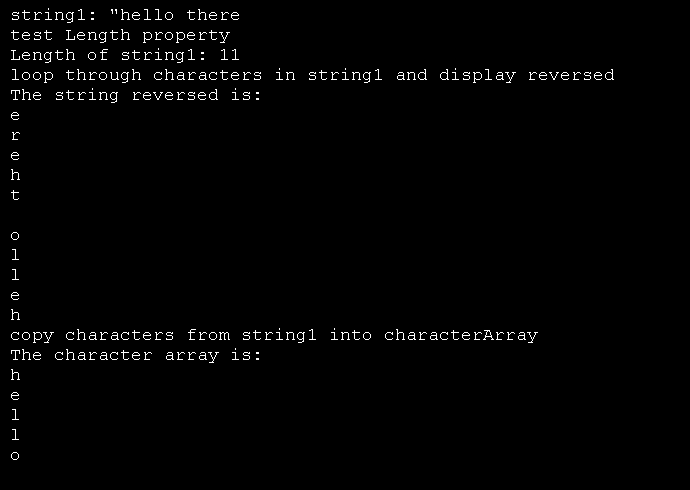 |
12. | Comparing strings | | 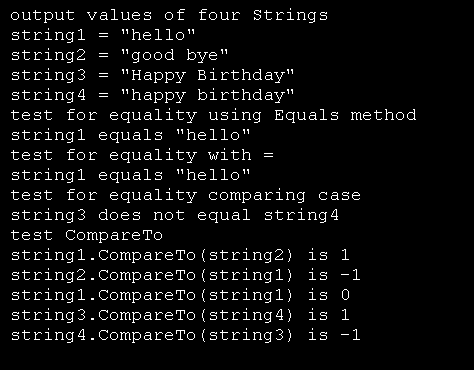 |
13. | Demonstrating StartsWith and EndsWith methods | | 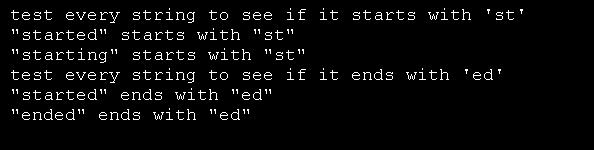 |
14. | String Substring method | |  |
15. | String class Concat method | |  |
16. | String ToLower and ToUpper | | 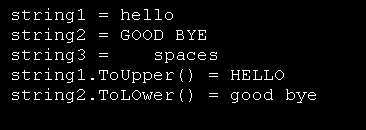 |
17. | String Trim method | | 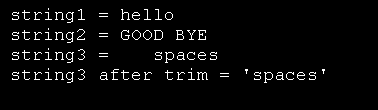 |
18. | String ToString method | | 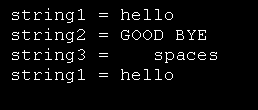 |
19. | String ToCharArray method | | 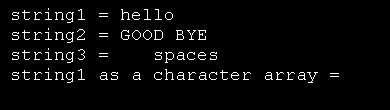 |
20. | Concatenate the strings and display the results | | 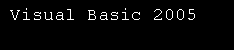 |
21. | The length of the string | |  |
22. | Display the first part of a string | | |
23. | String: set value and display | | |
24. | Concatenate the strings | | 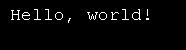 |
25. | Display the length of the string | | 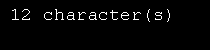 |
26. | Get sub string | |  |
27. | Replace the string occurance | | 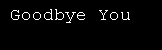 |
28. | String Replace Demo | | 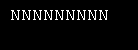 |
29. | Concatenate String with Integer into one String | |  |
30. | Concatenate Strings | |  |
31. | String Copy | | 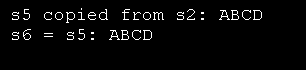 |
32. | String.Equals Demo | | 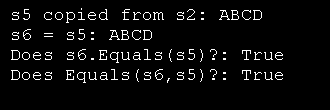 |
33. | Convert to Upper case and Lower Case | | 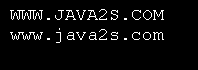 |
34. | Trim String space | |  |
35. | String ends with | |  |
36. | String concatenation | | |
37. | Create a string that consists of a character repeated 20 times | | |
38. | Append Date string to existing string | | |
39. | Extract the second word | | |
40. | String Class Represents text as a series of Unicode characters. | | |
41. | Create a string from a character array | | |
42. | Create a string that consists of a character repeated 20 times | | |
43. | Format Date with D | | |
44. | Append string together | | |
45. | Format a DateTime | | |
46. | Is Surrogate Pair | | |
47. | Is Punctuation and Is WhiteSpace | | |
48. | Create StreamWriter for Unicode encoding | | |
49. | Format Date by different Culture | | |
50. | Parse String to Date with various Culture settings | | |
51. | Perform a word sort using the current (en-US) culture | | |
52. | Perform a word sort using the invariant culture | | |
53. | Perform an ordinal sort | | |
54. | Perform a string sort using the current culture | | |
55. | Find string with different StringComparison | | |
56. | Culture-sensitive test for equality | | |
57. | Ordinal test for equality | | |
58. | String.Format Method (IFormatProvider, String, Object[]) | | |
59. | Format Date with different Culture | | |
60. | StringBuilder Class Represents a mutable string of characters | | |
61. | CultureInfo Class provides information about a specific culture | | |
62. | CultureInfo's English Name and | | |
63. | CultureInfo.CurrentCulture Property gets the CultureInfo that represents the culture used by the current thread. | | |
64. | Encoding Class represents a character encoding. | | |
65. | String.Chars Property gets the Char object at a specified position in the current String object. | | |
66. | String.Concat | | |
67. | String.Contains Method returns a value indicating whether the specified String object occurs within this string. | | |
68. | String.Copy | | 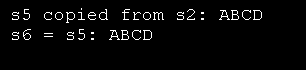 |
69. | String.CopyTo Method | | |
70. | String.EndsWith (String, StringComparison) | | |
71. | String.Equals Method | | |
72. | String.Equals (String) | | |
73. | String.Format (IFormatProvider, String, Object[]) | | |
74. | Customer Formatter | | |
75. | String.Format Method (String, Object) | | |
76. | String.Format (String, Object, Object) | | |
77. | String.GetEnumerator | | |
78. | String.GetHashCode Method | | |
79. | String.GetTypeCode Method returns the TypeCode for class String. | | |
80. | Sample for String.Intern(String) | | |
81. | String.IsNullOrEmpty | | |
82. | String.Join Method | | |
83. | Concatenates the members of a collection, using the specified separator between each member. | | |
84. | String.Length Property gets the number of characters in the current String object. | | |
85. | String.PadLeft Method (Int32) | | |
86. | String.PadLeft Method (Int32, Char) | | |
87. | String.PadRight | | |
88. | String.PadRight Method (Int32, Char) | | |
89. | String.Remove | | |
90. | Deletes a specified number of characters from this instance beginning at a specified position. | | |
91. | String.Replace (Char, Char) | | |
92. | String.Replace (String, String) | | |
93. | Retrieves a substring | | |
94. | Retrieves a substring which starts at a specified character position and has a specified length. | | |
95. | Copies the characters to a Unicode character array. | | |
96. | Convert to lowercase according to Culture settings | | |
97. | Convert string to lowercase | | |
98. | Convert String to lowercase using the casing rules of the invariant culture. | | |
99. | [Object].ReferenceEquals | | |
100. | String.ToUpper (CultureInfo) | | |
101. | String.ToUpper | | |
102. | Convert String to uppercase using the casing rules of the invariant culture. | | |
103. | Removes all leading and trailing occurrences of a set of characters | | |
104. | Removes all leading and trailing white-space characters from the current String object. | | |
105. | Removes all trailing occurrences of a set of characters | | |
106. | Encode the original string using the ASCII encoder | | |
107. | CharEnumerator Class supports iterating over a String | | |