Database Connection state change event
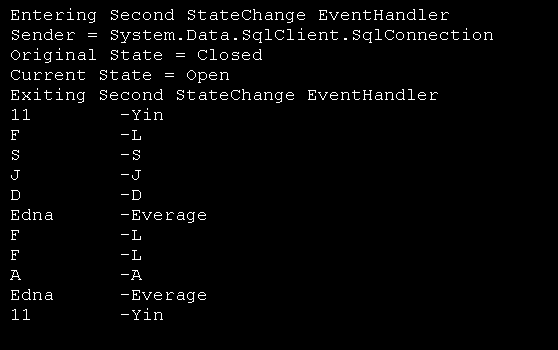
Imports System
Imports System.Data
Imports System.Data.SqlClient
public class MainClass
Shared Sub Main()
Dim thisConnection As New SqlConnection("server=(local)\SQLEXPRESS;" & _
"integrated security=sspi;database=MyDatabase")
' Sql Query
Dim sql As String = "SELECT FirstName, LastName From Employee"
' Create command
Dim thisCommand As New SqlCommand(sql, thisConnection)
' Add second handler to StateChange event
AddHandler thisConnection.StateChange, AddressOf StateIsChanged
Try
' Open connection and fire two statechange events
thisConnection.Open()
' Create datareader
Dim thisReader As SqlDataReader = thisCommand.ExecuteReader()
' Display rows in event log
While thisReader.Read()
Console.WriteLine(thisReader.GetString(0) _
+ "-" + thisReader.GetString(1))
End While
Catch ex As SqlException
Console.WriteLine(ex.Message)
Finally
' Remove second handler from StateChange event
RemoveHandler thisConnection.StateChange, AddressOf StateIsChanged
' Close connection and fire one StateChange event
thisConnection.Close()
End Try
End Sub
Shared Private Sub StateIsChanged(ByVal sender As Object, ByVal e As System.Data.StateChangeEventArgs)
' Event handler for the StateChange Event
Console.WriteLine("Entering Second StateChange EventHandler")
Console.WriteLine("Sender = " + sender.ToString())
Console.WriteLine("Original State = " + e.OriginalState.ToString())
Console.WriteLine("Current State = " + e.CurrentState.ToString())
Console.WriteLine("Exiting Second StateChange EventHandler")
End Sub
End Class
Related examples in the same category