1. | Create elements from one array to another array | | |
2. | Creates a one-dimensional Array of the specified Type and length, with zero-based indexing. | | |
3. | Creates a two-dimensional Array of the specified Type and dimension lengths, with zero-based indexing. | | |
4. | Creates a three-dimensional Array of the specified Type and dimension lengths, with zero-based indexing. | | |
5. | Creates a multidimensional Array of the specified Type and dimension lengths, with zero-based indexing. | | |
6. | Creates a multidimensional Array of the specified Type and dimension lengths, with the specified lower bounds. | | |
7. | Bounded array Example | |  |
8. | For Each loops through Array | | 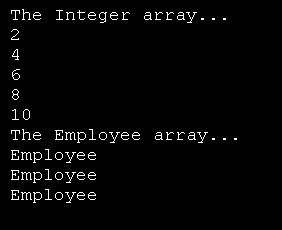 |
9. | Two ways to loop through Array | | 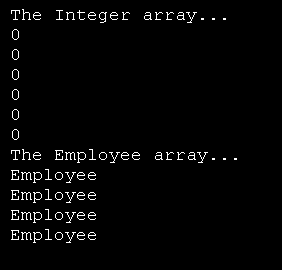 |
|
10. | A simple class to store in the array | | |
11. | Array UBound | |  |
12. | Set Array Element Value by Index | | |
13. | Array IndexOf and LastIndexOf | |  |
14. | Use Array CreateInstance to Create Array | |  |
15. | Array Performance Test: One-dimensional array | | 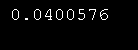 |
16. | Free array's memory | | |
17. | Array Performance Test: SetValue(i, i) | | 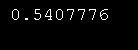 |
18. | Declaring, allocating and initializing arrays | | 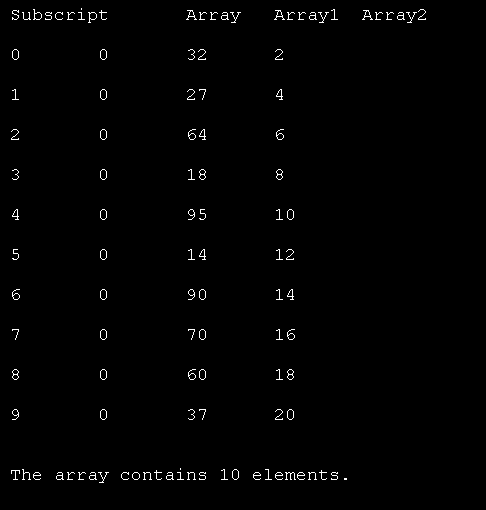 |
19. | Use For Each/Next to find a minimum grade | |  |
20. | Reference an Element in an Array by Index | |  |
21. | Array Upper Bound and Lower Bound | | 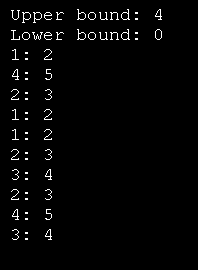 |
22. | Init an Array in Declaration | | 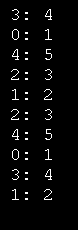 |
23. | Array Length Property | |  |
24. | Declare an Array and reference its member | |  |
25. | Store your won Class in an Array | |  |
26. | Array Class provides methods for creating, manipulating, searching, and sorting arrays | | |
27. | Copies the last two elements from the Object array to the integer array | | |
28. | Creates and initializes a new three-dimensional Array of type Int32. | | |
29. | Sets a range of elements in the Array to zero, to false, or to Nothing, depending on the element type. | | |
30. | Creates a shallow copy of the Array. | | |
31. | Converts an array of one type to an array of another type. | | |
32. | Copies a range of elements from an Array to another Array | | |
33. | Copies all the elements from one Array to another Array | | |
34. | Performs the specified action on each element of the specified array. | | |
35. | Returns an IEnumerator for the Array. | | |
36. | Reverses the sequence of the elements in the entire one-dimensional Array. | | |
37. | Array.GetLength | | |
38. | Gets the lower bound of the specified dimension in the Array. | | |
39. | Uses GetLowerBound and GetUpperBound in the for loop | | |
40. | Gets value at the specified position in the one-dimensional Array | | |
41. | Reverses the elements in a range | | |