Interface inherits interface
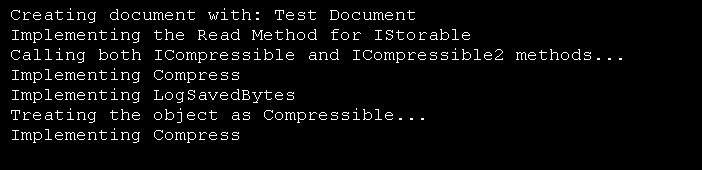
Imports System
Public Class MainClass
Shared Sub Main()
Dim doc As New Document("Test Document")
If TypeOf doc Is IStorable Then
Dim isDoc As IStorable = doc
isDoc.Read()
Else
Console.WriteLine("Could not cast to IStorable")
End If
If TypeOf doc Is ICompressible2 Then
Dim ilDoc As ICompressible2 = doc
Console.Write("Calling both ICompressible and ")
Console.WriteLine("ICompressible2 methods...")
ilDoc.Compress()
ilDoc.LogSavedBytes()
Else
Console.WriteLine("Could not cast to ICompressible2")
End If
If TypeOf doc Is ICompressible Then
Dim icDoc As ICompressible = doc
Console.WriteLine( _
"Treating the object as Compressible... ")
icDoc.Compress()
Else
Console.WriteLine("Could not cast to ICompressible")
End If
End Sub
End Class
Interface IStorable
Sub Read()
Sub Write(ByVal obj As Object)
Property Status() As Integer
End Interface
Interface ICompressible
Sub Compress()
Sub Decompress()
End Interface
Interface ICompressible2
Inherits ICompressible
Sub LogSavedBytes()
End Interface
Public Class Document
Implements ICompressible2, IStorable
Public Sub New(s As String)
Console.WriteLine("Creating document with: {0}", s)
End Sub
Public Sub Read() Implements IStorable.Read
Console.WriteLine("Implementing the Read Method for IStorable")
End Sub
Public Sub Write(ByVal o As Object) Implements IStorable.Write
Console.WriteLine( _
"Implementing the Write Method for IStorable")
End Sub
Public Property Status() As Integer Implements IStorable.Status
Get
Return myStatus
End Get
Set(ByVal Value As Integer)
myStatus = Value
End Set
End Property
Public Sub Compress() Implements ICompressible.Compress
Console.WriteLine("Implementing Compress")
End Sub
Public Sub Decompress() Implements ICompressible.Decompress
Console.WriteLine("Implementing Decompress")
End Sub
Public Sub LogSavedBytes() Implements ICompressible2.LogSavedBytes
Console.WriteLine("Implementing LogSavedBytes")
End Sub
Private myStatus As Integer = 0
End Class
Related examples in the same category