Define and use Interface Age
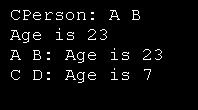
Imports System
Imports System.Windows.Forms
Imports System.Drawing.Text
Imports System.Drawing
Imports System.Drawing.Drawing2D
Public Class MainClass
Shared Sub Main(ByVal args As String())
Dim person As New CPerson("A", "B", 1983)
Dim iAgeArray As IAge() = New IAge(1) {}
iAgeArray(0) = person
iAgeArray(1) = New CPerson("C", "D", 1999)
Console.WriteLine( person.ToString() & ": " & _
person.Name & vbCrLf & "Age is " & person.Age )
Dim ageReference As IAge
For Each ageReference In iAgeArray
Console.WriteLine( ageReference.Name & ": " & _
"Age is " & ageReference.Age )
Next
End Sub
End Class
Public Interface IAge
ReadOnly Property Age() As Integer
ReadOnly Property Name() As String
End Interface
Public Class CPerson
Implements IAge
Private mYearBorn As Integer
Private mFirstName As String
Private mLastName As String
Public Sub New(ByVal firstNameValue As String, _
ByVal lastNameValue As String, _
ByVal yearBornValue As Integer)
mFirstName = firstNameValue
mLastName = lastNameValue
If (yearBornValue > 0 AndAlso _
yearBornValue <= Date.Now.Year) Then
mYearBorn = yearBornValue
Else
mYearBorn = Date.Now.Year
End If
End Sub
ReadOnly Property Age() As Integer _
Implements IAge.Age
Get
Return Date.Now.Year - mYearBorn
End Get
End Property
ReadOnly Property Name() As String _
Implements IAge.Name
Get
Return mFirstName & " " & mLastName
End Get
End Property
End Class
Related examples in the same category