PointFromTo With Code
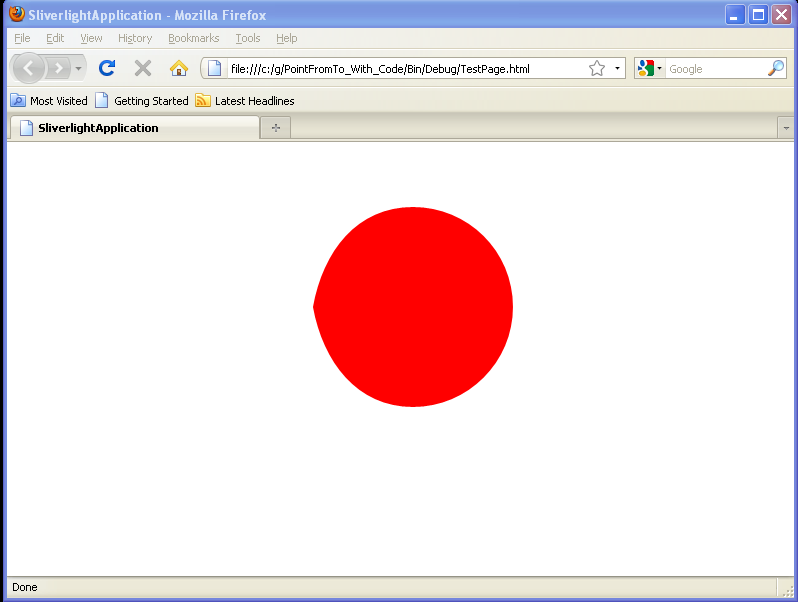
<UserControl x:Class='SilverlightApplication3.MainPage'
xmlns='http://schemas.microsoft.com/winfx/2006/xaml/presentation'
xmlns:x='http://schemas.microsoft.com/winfx/2006/xaml'
xmlns:d='http://schemas.microsoft.com/expression/blend/2008'
xmlns:mc='http://schemas.openxmlformats.org/markup-compatibility/2006'
mc:Ignorable='d'
d:DesignWidth='640' d:DesignHeight='480'>
<Canvas x:Name="LayoutRoot" Background="White" Visibility="Visible">
<Path Height="277" Width="200" Canvas.Left="306" Canvas.Top="65" Fill="#FFFF0000" Stretch="None" x:Name="RedPath" Cursor="Hand">
<Path.Data>
<PathGeometry>
<PathFigure IsClosed="True" StartPoint="200,100">
<BezierSegment Point1="200,155" Point2="155,200" Point3="100,200"/>
<BezierSegment Point1="44,200" Point2="10,155" Point3="0,100"/>
<BezierSegment Point1="10,44" Point2="44,0" Point3="100,0"/>
<BezierSegment Point1="155,0" Point2="200,44" Point3="200,100"/>
</PathFigure>
</PathGeometry>
</Path.Data>
</Path>
</Canvas>
</UserControl>
//File: Page.xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace SilverlightApplication3
{
public partial class MainPage : UserControl
{
private Storyboard MovePointDown = new Storyboard();
private Storyboard MovePointUp = new Storyboard();
private PointAnimation P1Anim = new PointAnimation();
private PointAnimation P2Anim = new PointAnimation();
private PointAnimation P3Anim = new PointAnimation();
private PointAnimation P1AnimBack = new PointAnimation();
private PointAnimation P2AnimBack = new PointAnimation();
private PointAnimation P3AnimBack = new PointAnimation();
public MainPage()
{
InitializeComponent();
Storyboard.SetTargetName(MovePointDown, "RedPath");
Storyboard.SetTargetName(MovePointUp, "RedPath");
P1Anim.SetValue(Storyboard.TargetPropertyProperty, new PropertyPath("(Path.Data).(PathGeometry.Figures)[0].(PathFigure.Segments)[1].(BezierSegment.Point1)"));
P1Anim.Duration = new Duration(TimeSpan.FromSeconds(0.5));
P1Anim.To = new Point(45, 277);
MovePointDown.Children.Add(P1Anim);
P2Anim.SetValue(Storyboard.TargetPropertyProperty, new PropertyPath("(Path.Data).(PathGeometry.Figures)[0].(PathFigure.Segments)[0].(BezierSegment.Point2)"));
P2Anim.Duration = new Duration(TimeSpan.FromSeconds(0.5));
P2Anim.To = new Point(155, 277);
MovePointDown.Children.Add(P2Anim);
P3Anim.SetValue(Storyboard.TargetPropertyProperty, new PropertyPath("(Path.Data).(PathGeometry.Figures)[0].(PathFigure.Segments)[0].(BezierSegment.Point3)"));
P3Anim.Duration = new Duration(TimeSpan.FromSeconds(0.5));
P3Anim.To = new Point(100, 277);
MovePointDown.Children.Add(P3Anim);
P1AnimBack.SetValue(Storyboard.TargetPropertyProperty, new PropertyPath("(Path.Data).(PathGeometry.Figures)[0].(PathFigure.Segments)[1].(BezierSegment.Point1)"));
P1AnimBack.Duration = new Duration(TimeSpan.FromSeconds(0.5));
P1AnimBack.To = new Point(45, 200);
MovePointUp.Children.Add(P1AnimBack);
P2AnimBack.SetValue(Storyboard.TargetPropertyProperty, new PropertyPath("(Path.Data).(PathGeometry.Figures)[0].(PathFigure.Segments)[0].(BezierSegment.Point2)"));
P2AnimBack.Duration = new Duration(TimeSpan.FromSeconds(0.5));
P2AnimBack.To = new Point(155, 200);
MovePointUp.Children.Add(P2AnimBack);
P3AnimBack.SetValue(Storyboard.TargetPropertyProperty, new PropertyPath("(Path.Data).(PathGeometry.Figures)[0].(PathFigure.Segments)[0].(BezierSegment.Point3)"));
P3AnimBack.Duration = new Duration(TimeSpan.FromSeconds(0.5));
P3AnimBack.To = new Point(100, 200);
MovePointUp.Children.Add(P3AnimBack);
LayoutRoot.Resources.Add("MovePointDown", MovePointDown);
LayoutRoot.Resources.Add("MovePointUp", MovePointUp);
RedPath.MouseEnter += new MouseEventHandler(RedPath_MouseEnter);
RedPath.MouseLeave += new MouseEventHandler(RedPath_MouseLeave);
}
void RedPath_MouseLeave(object sender, MouseEventArgs e)
{
MovePointUp.Begin();
}
void RedPath_MouseEnter(object sender, MouseEventArgs e)
{
MovePointDown.Begin();
}
}
}
Related examples in the same category