This example demonstrates the use of JButton, JTextField and JLabel
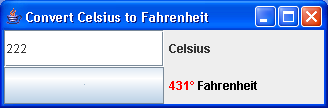
/* From http://java.sun.com/docs/books/tutorial/index.html */
/*
* Copyright (c) 2006 Sun Microsystems, Inc. All Rights Reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* -Redistribution of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* -Redistribution in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* Neither the name of Sun Microsystems, Inc. or the names of contributors may
* be used to endorse or promote products derived from this software without
* specific prior written permission.
*
* This software is provided "AS IS," without a warranty of any kind. ALL
* EXPRESS OR IMPLIED CONDITIONS, REPRESENTATIONS AND WARRANTIES, INCLUDING
* ANY IMPLIED WARRANTY OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE
* OR NON-INFRINGEMENT, ARE HEREBY EXCLUDED. SUN MIDROSYSTEMS, INC. ("SUN")
* AND ITS LICENSORS SHALL NOT BE LIABLE FOR ANY DAMAGES SUFFERED BY LICENSEE
* AS A RESULT OF USING, MODIFYING OR DISTRIBUTING THIS SOFTWARE OR ITS
* DERIVATIVES. IN NO EVENT WILL SUN OR ITS LICENSORS BE LIABLE FOR ANY LOST
* REVENUE, PROFIT OR DATA, OR FOR DIRECT, INDIRECT, SPECIAL, CONSEQUENTIAL,
* INCIDENTAL OR PUNITIVE DAMAGES, HOWEVER CAUSED AND REGARDLESS OF THE THEORY
* OF LIABILITY, ARISING OUT OF THE USE OF OR INABILITY TO USE THIS SOFTWARE,
* EVEN IF SUN HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES.
*
* You acknowledge that this software is not designed, licensed or intended
* for use in the design, construction, operation or maintenance of any
* nuclear facility.
*/
// v 1.3
import java.awt.BorderLayout;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.BorderFactory;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.SwingConstants;
import javax.swing.UIManager;
// This example demonstrates the use of JButton, JTextField and JLabel.
public class CelsiusConverter2 implements ActionListener {
JFrame converterFrame;
JPanel converterPanel;
JTextField tempCelsius;
JLabel celsiusLabel, fahrenheitLabel;
JButton convertTemp;
// Constructor
public CelsiusConverter2() {
// Create the frame and container.
converterFrame = new JFrame("Convert Celsius to Fahrenheit");
converterPanel = new JPanel();
converterPanel.setLayout(new GridLayout(2, 2));
// Add the widgets.
addWidgets();
// Add the panel to the frame.
converterFrame.getContentPane()
.add(converterPanel, BorderLayout.CENTER);
// Exit when the window is closed.
converterFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Show the converter.
converterFrame.pack();
converterFrame.setVisible(true);
}
// Create and add the widgets for converter.
private void addWidgets() {
// Create widgets.
ImageIcon icon = new ImageIcon("images/convert.gif",
"Convert temperature");
tempCelsius = new JTextField(2);
celsiusLabel = new JLabel("Celsius", SwingConstants.LEFT);
convertTemp = new JButton(icon);
fahrenheitLabel = new JLabel("Fahrenheit", SwingConstants.LEFT);
celsiusLabel.setBorder(BorderFactory.createEmptyBorder(5, 5, 5, 5));
fahrenheitLabel.setBorder(BorderFactory.createEmptyBorder(5, 5, 5, 5));
// Listen to events from Convert button.
convertTemp.addActionListener(this);
// Add widgets to container.
converterPanel.add(tempCelsius);
converterPanel.add(celsiusLabel);
converterPanel.add(convertTemp);
converterPanel.add(fahrenheitLabel);
}
// Implementation of ActionListener interface.
public void actionPerformed(ActionEvent event) {
// Parse degrees Celsius as a double and convert to Fahrenheit.
int tempFahr = (int) ((Double.parseDouble(tempCelsius.getText())) * 1.8 + 32);
// Set fahrenheitLabel to new value and set font color based on the
// temperature.
if (tempFahr <= 32) {
fahrenheitLabel
.setText("<html><Font Color=blue>"
+ tempFahr
+ "° </Font><Font Color=black> Fahrenheit</font></html>");
} else if (tempFahr <= 80) {
fahrenheitLabel
.setText("<html><Font Color=green>"
+ tempFahr
+ "° </Font><Font Color=black> Fahrenheit </Font></html>");
} else {
fahrenheitLabel
.setText("<html><Font Color=red>"
+ tempFahr
+ "° </Font><Font Color=black> Fahrenheit</Font></html>");
}
}
// main method
public static void main(String[] args) {
// set the look and feel
try {
UIManager.setLookAndFeel(UIManager
.getCrossPlatformLookAndFeelClassName());
} catch (Exception e) {
}
CelsiusConverter2 converter = new CelsiusConverter2();
}
}
Related examples in the same category