Table model is based on call back
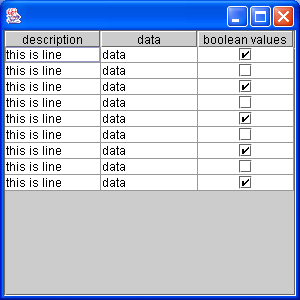
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.table.AbstractTableModel;
public class ExampleTableModel extends AbstractTableModel {
String columnName[] = { "description", "data", "boolean values" };
Class columnType[] = { String.class, String.class, Boolean.class };
Object table[][] = { { "this is line", "data", new Boolean(true) },
{ "this is line", "data", new Boolean(false) },
{ "this is line", "data", new Boolean(true) },
{ "this is line", "data", new Boolean(false) },
{ "this is line", "data", new Boolean(true) },
{ "this is line", "data", new Boolean(false) },
{ "this is line", "data", new Boolean(true) },
{ "this is line", "data", new Boolean(false) },
{ "this is line", "data", new Boolean(true) } };
public int getColumnCount() {
return table[0].length;
}
public int getRowCount() {
return table.length;
}
public Object getValueAt(int r, int c) {
return table[r][c];
}
public String getColumnName(int column) {
return columnName[column];
}
public Class getColumnClass(int c) {
return columnType[c];
}
public boolean isCellEditable(int r, int c) {
return false;
}
public void setValueAt(Object aValue, int r, int c) {
}
public static void main(String[] arg){
JFrame f = new JFrame();
f.getContentPane().add(new JScrollPane(new JTable(new ExampleTableModel())));
f.setSize(300,300);
f.show();
}
}
Related examples in the same category