Custom table model, File data based Model
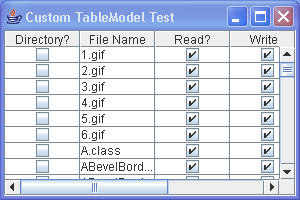
/*
Java Swing, 2nd Edition
By Marc Loy, Robert Eckstein, Dave Wood, James Elliott, Brian Cole
ISBN: 0-596-00408-7
Publisher: O'Reilly
*/
// FileTable2.java
//A test frame for the custom table model, FileModel. This version uses a
//custom renderer (BigRenderer.java) to flag large files with an exclamation
//point icon.
import java.awt.BorderLayout;
import java.awt.Component;
import java.io.File;
import java.util.Date;
import javax.swing.Icon;
import javax.swing.ImageIcon;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.SwingConstants;
import javax.swing.table.AbstractTableModel;
import javax.swing.table.DefaultTableCellRenderer;
public class FileTable2 extends JFrame {
public FileTable2() {
super("Custom TableModel Test");
setSize(300, 200);
setDefaultCloseOperation(EXIT_ON_CLOSE);
FileModel fm = new FileModel();
JTable jt = new JTable(fm);
jt.setAutoResizeMode(JTable.AUTO_RESIZE_OFF);
jt.setColumnSelectionAllowed(true);
jt.setDefaultRenderer(Number.class, new BigRenderer(1000));
JScrollPane jsp = new JScrollPane(jt);
getContentPane().add(jsp, BorderLayout.CENTER);
}
public static void main(String args[]) {
FileTable2 ft = new FileTable2();
ft.setVisible(true);
}
}
//FileModel.java
//A custom table model to display information on a directory of files.
//
class FileModel extends AbstractTableModel {
String titles[] = new String[] { "Directory?", "File Name", "Read?",
"Write?", "Size", "Last Modified" };
Class types[] = new Class[] { Boolean.class, String.class, Boolean.class,
Boolean.class, Number.class, Date.class };
Object data[][];
public FileModel() {
this(".");
}
public FileModel(String dir) {
File pwd = new File(dir);
setFileStats(pwd);
}
// Implement the methods of the TableModel interface we're interested
// in. Only getRowCount(), getColumnCount() and getValueAt() are
// required. The other methods tailor the look of the table.
public int getRowCount() {
return data.length;
}
public int getColumnCount() {
return titles.length;
}
public String getColumnName(int c) {
return titles[c];
}
public Class getColumnClass(int c) {
return types[c];
}
public Object getValueAt(int r, int c) {
return data[r][c];
}
// Our own method for setting/changing the current directory
// being displayed. This method fills the data set with file info
// from the given directory. It also fires an update event so this
// method could also be called after the table is on display.
public void setFileStats(File dir) {
String files[] = dir.list();
data = new Object[files.length][titles.length];
for (int i = 0; i < files.length; i++) {
File tmp = new File(files[i]);
data[i][0] = new Boolean(tmp.isDirectory());
data[i][1] = tmp.getName();
data[i][2] = new Boolean(tmp.canRead());
data[i][3] = new Boolean(tmp.canWrite());
data[i][4] = new Long(tmp.length());
data[i][5] = new Date(tmp.lastModified());
}
// Just in case anyone's listening...
fireTableDataChanged();
}
}
//BigRenderer.java
//A renderer for numbers that shows an icon in front of big numbers.
//
class BigRenderer extends DefaultTableCellRenderer {
double threshold;
Icon bang = new ImageIcon("bang.gif");
public BigRenderer(double t) {
threshold = t;
setHorizontalAlignment(JLabel.RIGHT);
setHorizontalTextPosition(SwingConstants.RIGHT);
}
public Component getTableCellRendererComponent(JTable table, Object value,
boolean isSelected, boolean hasFocus, int row, int col) {
// be a little paranoid about where the user tries to use this renderer
if (value instanceof Number) {
if (((Number) value).doubleValue() > threshold) {
setIcon(bang);
} else {
setIcon(null);
}
} else {
setIcon(null);
}
return super.getTableCellRendererComponent(table, value, isSelected,
hasFocus, row, col);
}
}
Related examples in the same category