Simple GUI demo of UndoManager and friends
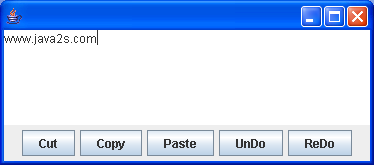
/*
* Copyright (c) Ian F. Darwin, http://www.darwinsys.com/, 1996-2002.
* All rights reserved. Software written by Ian F. Darwin and others.
* $Id: LICENSE,v 1.8 2004/02/09 03:33:38 ian Exp $
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE AUTHOR AND CONTRIBUTORS ``AS IS''
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED
* TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHOR OR CONTRIBUTORS
* BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* Java, the Duke mascot, and all variants of Sun's Java "steaming coffee
* cup" logo are trademarks of Sun Microsystems. Sun's, and James Gosling's,
* pioneering role in inventing and promulgating (and standardizing) the Java
* language and environment is gratefully acknowledged.
*
* The pioneering role of Dennis Ritchie and Bjarne Stroustrup, of AT&T, for
* inventing predecessor languages C and C++ is also gratefully acknowledged.
*/
import java.awt.BorderLayout;
import java.awt.Container;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextArea;
import javax.swing.undo.UndoManager;
/**
* Simple GUI demo of UndoManager and friends.
*
* @author Ian Darwin http://www.darwinsys.com/
*/
public class UndoDemo extends JFrame {
JTextArea ta;
UndoManager um;
/** Simple main program that just constructs and shows the GUI */
public static void main(String[] files) {
new UndoDemo().setVisible(true);
}
/** Construct a GUI that demonstrates use of UndoManager */
public UndoDemo() {
Container cp = getContentPane();
cp.add(ta = new JTextArea(20, 60), BorderLayout.CENTER);
JPanel bp;
cp.add(bp = new JPanel(), BorderLayout.SOUTH);
// Create a javax.swing.undo.UndoManager; this is an amazing class that
// keeps a Stack of UndoableEdits and lets you invoke them;
// by registering it as a Listener on the TextComponent.Document,
// the Document will create the UndoableEdit objects and send them
// to the UndoManager. Between them they do ALL the work!
um = new UndoManager();
ta.getDocument().addUndoableEditListener(um);
// Create the buttons
JButton cutButton, copyButton, pasteButton, undoButton, redoButton;
bp.add(cutButton = new JButton("Cut"));
cutButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
ta.cut();
}
});
bp.add(copyButton = new JButton("Copy"));
copyButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
ta.copy();
}
});
bp.add(pasteButton = new JButton("Paste"));
pasteButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
ta.paste();
}
});
bp.add(undoButton = new JButton("UnDo"));
undoButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if (um.canUndo()) {
um.undo();
} else {
warn("Can't undo");
}
}
});
bp.add(redoButton = new JButton("ReDo"));
redoButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if (um.canRedo()) {
um.redo();
} else {
warn("Can't redo");
}
}
});
pack();
setDefaultCloseOperation(EXIT_ON_CLOSE);
}
void warn(String msg) {
JOptionPane.showMessageDialog(this, "Warning: " + msg, "Warning",
JOptionPane.WARNING_MESSAGE);
}
}
Related examples in the same category