Actions MenuBar
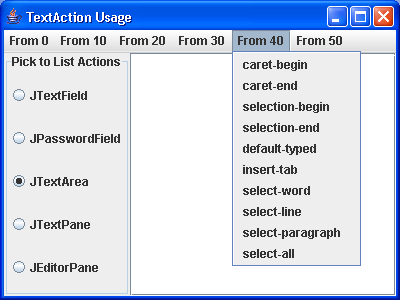
/*
Definitive Guide to Swing for Java 2, Second Edition
By John Zukowski
ISBN: 1-893115-78-X
Publisher: APress
*/
import java.awt.BorderLayout;
import java.awt.Component;
import java.awt.Container;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.ItemListener;
import java.util.Enumeration;
import java.util.Vector;
import javax.swing.AbstractButton;
import javax.swing.Action;
import javax.swing.BorderFactory;
import javax.swing.ButtonGroup;
import javax.swing.JEditorPane;
import javax.swing.JFrame;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JRadioButton;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.JTextField;
import javax.swing.JTextPane;
import javax.swing.border.Border;
import javax.swing.event.ChangeListener;
import javax.swing.text.JTextComponent;
public class ActionsMenuBar {
public static void main(String args[]) {
final JFrame frame = new JFrame("TextAction Usage");
Container contentPane = frame.getContentPane();
final JScrollPane scrollPane = new JScrollPane();
contentPane.add(scrollPane, BorderLayout.CENTER);
final JMenuBar menuBar = new JMenuBar();
frame.setJMenuBar(menuBar);
ActionListener actionListener = new ActionListener() {
JTextComponent component;
public void actionPerformed(ActionEvent actionEvent) {
// Determine which component selected
String command = actionEvent.getActionCommand();
if (command.equals("JTextField")) {
component = new JTextField();
} else if (command.equals("JPasswordField")) {
component = new JPasswordField();
} else if (command.equals("JTextArea")) {
component = new JTextArea();
} else if (command.equals("JTextPane")) {
component = new JTextPane();
} else {
component = new JEditorPane();
}
scrollPane.setViewportView(component);
// Process action list
Action actions[] = component.getActions();
menuBar.removeAll();
menuBar.revalidate();
JMenu menu = null;
for (int i = 0, n = actions.length; i < n; i++) {
if ((i % 10) == 0) {
menu = new JMenu("From " + i);
menuBar.add(menu);
}
menu.add(actions[i]);
}
menuBar.revalidate();
}
};
String components[] = { "JTextField", "JPasswordField", "JTextArea",
"JTextPane", "JEditorPane" };
final Container componentsContainer = RadioButtonUtils
.createRadioButtonGrouping(components, "Pick to List Actions",
actionListener);
contentPane.add(componentsContainer, BorderLayout.WEST);
frame.setSize(400, 300);
frame.setVisible(true);
}
}
class RadioButtonUtils {
private RadioButtonUtils() {
// private constructor so you can't create instances
}
public static Enumeration getSelectedElements(Container container) {
Vector selections = new Vector();
Component components[] = container.getComponents();
for (int i = 0, n = components.length; i < n; i++) {
if (components[i] instanceof AbstractButton) {
AbstractButton button = (AbstractButton) components[i];
if (button.isSelected()) {
selections.addElement(button.getText());
}
}
}
return selections.elements();
}
public static Container createRadioButtonGrouping(String elements[]) {
return createRadioButtonGrouping(elements, null, null, null, null);
}
public static Container createRadioButtonGrouping(String elements[],
String title) {
return createRadioButtonGrouping(elements, title, null, null, null);
}
public static Container createRadioButtonGrouping(String elements[],
String title, ItemListener itemListener) {
return createRadioButtonGrouping(elements, title, null, itemListener,
null);
}
public static Container createRadioButtonGrouping(String elements[],
String title, ActionListener actionListener) {
return createRadioButtonGrouping(elements, title, actionListener, null,
null);
}
public static Container createRadioButtonGrouping(String elements[],
String title, ActionListener actionListener,
ItemListener itemListener) {
return createRadioButtonGrouping(elements, title, actionListener,
itemListener, null);
}
public static Container createRadioButtonGrouping(String elements[],
String title, ActionListener actionListener,
ItemListener itemListener, ChangeListener changeListener) {
JPanel panel = new JPanel(new GridLayout(0, 1));
// If title set, create titled border
if (title != null) {
Border border = BorderFactory.createTitledBorder(title);
panel.setBorder(border);
}
// Create group
ButtonGroup group = new ButtonGroup();
JRadioButton aRadioButton;
// For each String passed in:
// Create button, add to panel, and add to group
for (int i = 0, n = elements.length; i < n; i++) {
aRadioButton = new JRadioButton(elements[i]);
panel.add(aRadioButton);
group.add(aRadioButton);
if (actionListener != null) {
aRadioButton.addActionListener(actionListener);
}
if (itemListener != null) {
aRadioButton.addItemListener(itemListener);
}
if (changeListener != null) {
aRadioButton.addChangeListener(changeListener);
}
}
return panel;
}
}
Related examples in the same category