Passive TextField 3
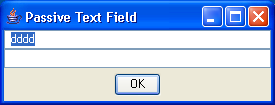
/*
Core SWING Advanced Programming
By Kim Topley
ISBN: 0 13 083292 8
Publisher: Prentice Hall
*/
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import javax.swing.BoxLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.UIManager;
import javax.swing.text.Document;
public class PassiveTextField extends JTextField {
public PassiveTextField() {
this(null, null, 0);
}
public PassiveTextField(String text) {
this(null, text, 0);
}
public PassiveTextField(int columns) {
this(null, null, columns);
}
public PassiveTextField(String text, int columns) {
this(null, text, columns);
}
public PassiveTextField(Document doc, String text, int columns) {
super(doc, text, columns);
}
public void processComponentKeyEvent(KeyEvent evt) {
switch (evt.getID()) {
case KeyEvent.KEY_PRESSED:
case KeyEvent.KEY_RELEASED:
if (evt.getKeyCode() == KeyEvent.VK_ENTER) {
return;
}
break;
case KeyEvent.KEY_TYPED:
if (evt.getKeyChar() == '\r') {
return;
}
break;
}
super.processComponentKeyEvent(evt);
}
// Test method
public static void main(String[] args) {
try {
UIManager.setLookAndFeel("com.sun.java.swing.plaf.windows.WindowsLookAndFeel");
} catch (Exception evt) {}
JFrame f = new JFrame("Passive Text Field");
f.getContentPane().setLayout(
new BoxLayout(f.getContentPane(), BoxLayout.Y_AXIS));
final PassiveTextField ptf = new PassiveTextField(32);
JTextField tf = new JTextField(32);
JPanel p = new JPanel();
JButton b = new JButton("OK");
p.add(b);
f.getContentPane().add(ptf);
f.getContentPane().add(tf);
f.getContentPane().add(p);
ActionListener l = new ActionListener() {
public void actionPerformed(ActionEvent evt) {
System.out.println("Action event from a text field");
}
};
ptf.addActionListener(l);
tf.addActionListener(l);
// Make the button the default button
f.getRootPane().setDefaultButton(b);
b.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent evt) {
System.out.println("Content of text field: <" + ptf.getText()
+ ">");
}
});
f.pack();
f.setVisible(true);
}
}
Related examples in the same category