Passive TextField 2
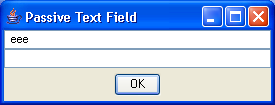
/*
Core SWING Advanced Programming
By Kim Topley
ISBN: 0 13 083292 8
Publisher: Prentice Hall
*/
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.Action;
import javax.swing.BoxLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.KeyStroke;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
import javax.swing.text.Document;
import javax.swing.text.Keymap;
public class PassiveTextField2 extends JTextField {
public PassiveTextField2() {
this(null, null, 0);
}
public PassiveTextField2(String text) {
this(null, text, 0);
}
public PassiveTextField2(int columns) {
this(null, null, columns);
}
public PassiveTextField2(String text, int columns) {
this(null, text, columns);
}
public PassiveTextField2(Document doc, String text, int columns) {
super(doc, text, columns);
}
public void setKeymap(Keymap map) {
if (map == null) {
// Uninstalling keymap.
super.setKeymap(null);
sharedKeymap = null;
return;
}
if (getKeymap() == null) {
if (sharedKeymap == null) {
// Initial keymap, or first
// keymap after L&F switch.
// Generate a new keymap
sharedKeymap = addKeymap(null, map.getResolveParent());
KeyStroke[] strokes = map.getBoundKeyStrokes();
for (int i = 0; i < strokes.length; i++) {
Action a = map.getAction(strokes[i]);
if (a.getValue(Action.NAME) == JTextField.notifyAction) {
continue;
}
sharedKeymap.addActionForKeyStroke(strokes[i], a);
}
}
map = sharedKeymap;
}
super.setKeymap(map);
}
protected static Keymap sharedKeymap;
// Test method
public static void main(String[] args) {
try {
UIManager.setLookAndFeel("com.sun.java.swing.plaf.windows.WindowsLookAndFeel");
} catch (Exception evt) {}
JFrame f = new JFrame("Passive Text Field");
f.getContentPane().setLayout(
new BoxLayout(f.getContentPane(), BoxLayout.Y_AXIS));
final PassiveTextField2 ptf = new PassiveTextField2(32);
JTextField tf = new JTextField(32);
JPanel p = new JPanel();
JButton b = new JButton("OK");
p.add(b);
f.getContentPane().add(ptf);
f.getContentPane().add(tf);
f.getContentPane().add(p);
SwingUtilities.updateComponentTreeUI(f);
ActionListener l = new ActionListener() {
public void actionPerformed(ActionEvent evt) {
System.out.println("Action event from a text field");
}
};
ptf.addActionListener(l);
tf.addActionListener(l);
// Make the button the default button
f.getRootPane().setDefaultButton(b);
b.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent evt) {
System.out.println("Content of text field: <" + ptf.getText()
+ ">");
}
});
f.pack();
f.setVisible(true);
}
}
Related examples in the same category