JScrollPane: Button Corner Sample
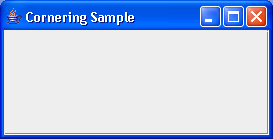
import java.awt.BorderLayout;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.Icon;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JScrollBar;
import javax.swing.JScrollPane;
public class ButtonCornerSample {
public static void main(String args[]) {
JFrame frame = new JFrame("Cornering Sample");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Icon acrossLogo = new ImageIcon("logo-across.jpg");
Icon downLogo = new ImageIcon("logo-down.jpg");
Icon bookCover = new ImageIcon("puzzlebook.jpg");
JLabel columnLabel = new JLabel(acrossLogo);
JLabel rowLabel = new JLabel(downLogo);
JLabel coverLabel = new JLabel(bookCover);
JButton button = new JButton("+");
button.setFont(new Font("Monospaced", Font.PLAIN, 8));
JScrollPane scrollPane = new JScrollPane(coverLabel);
scrollPane.setCorner(JScrollPane.UPPER_LEFT_CORNER, button);
scrollPane.setColumnHeaderView(columnLabel);
scrollPane.setRowHeaderView(rowLabel);
ActionListener actionListener = new JScrollPaneToTopAction(scrollPane);
button.addActionListener(actionListener);
frame.getContentPane().add(scrollPane, BorderLayout.CENTER);
frame.setSize(300, 200);
frame.setVisible(true);
}
}
class JScrollPaneToTopAction implements ActionListener {
JScrollPane scrollPane;
public JScrollPaneToTopAction(JScrollPane scrollPane) {
if (scrollPane == null) {
throw new IllegalArgumentException(
"JScrollPaneToTopAction: null JScrollPane");
}
this.scrollPane = scrollPane;
}
public void actionPerformed(ActionEvent actionEvent) {
JScrollBar verticalScrollBar = scrollPane.getVerticalScrollBar();
JScrollBar horizontalScrollBar = scrollPane.getHorizontalScrollBar();
verticalScrollBar.setValue(verticalScrollBar.getMinimum());
horizontalScrollBar.setValue(horizontalScrollBar.getMinimum());
}
}
Related examples in the same category