Demonstrating the MenuListener
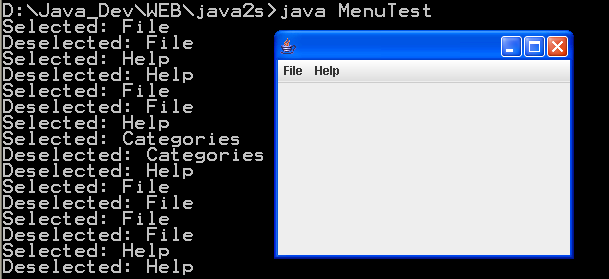
import javax.swing.ButtonGroup;
import javax.swing.JCheckBoxMenuItem;
import javax.swing.JFrame;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JRadioButtonMenuItem;
import javax.swing.event.MenuEvent;
import javax.swing.event.MenuListener;
public class MenuTest extends JFrame {
public MenuTest() {
super();
MenuListener listener = new MenuListener() {
public void menuCanceled(MenuEvent e) {
dumpInfo("Canceled", e);
}
public void menuDeselected(MenuEvent e) {
dumpInfo("Deselected", e);
}
public void menuSelected(MenuEvent e) {
dumpInfo("Selected", e);
}
private void dumpInfo(String s, MenuEvent e) {
JMenu menu = (JMenu) e.getSource();
System.out.println(s + ": " + menu.getText());
}
};
JMenu fileMenu = new JMenu("File");
fileMenu.addMenuListener(listener);
fileMenu.add(new JMenuItem("Open"));
fileMenu.add(new JMenuItem("Close"));
fileMenu.add(new JMenuItem("Exit"));
JMenu helpMenu = new JMenu("Help");
helpMenu.addMenuListener(listener);
helpMenu.add(new JMenuItem("About MenuTest"));
helpMenu.add(new JMenuItem("Class Hierarchy"));
helpMenu.addSeparator();
helpMenu.add(new JCheckBoxMenuItem("Balloon Help"));
JMenu subMenu = new JMenu("Categories");
subMenu.addMenuListener(listener);
JRadioButtonMenuItem rb;
ButtonGroup group = new ButtonGroup();
subMenu.add(rb = new JRadioButtonMenuItem("A Little Help", true));
group.add(rb);
subMenu.add(rb = new JRadioButtonMenuItem("A Lot of Help"));
group.add(rb);
helpMenu.add(subMenu);
JMenuBar mb = new JMenuBar();
mb.add(fileMenu);
mb.add(helpMenu);
setJMenuBar(mb);
}
public static void main(String args[]) {
JFrame frame = new MenuTest();
frame.setSize(300, 300);
frame.show();
}
}
Related examples in the same category