A frame with a panel for testing various exceptions
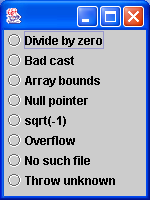
/*
This program is a part of the companion code for Core Java 8th ed.
(http://horstmann.com/corejava)
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
import java.awt.EventQueue;
import java.awt.event.*;
import javax.swing.*;
import java.io.*;
/**
* @version 1.33 2007-06-12
* @author Cay Horstmann
*/
public class ExceptTest
{
public static void main(String[] args)
{
EventQueue.invokeLater(new Runnable()
{
public void run()
{
ExceptTestFrame frame = new ExceptTestFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
});
}
}
/**
* A frame with a panel for testing various exceptions
*/
class ExceptTestFrame extends JFrame
{
public ExceptTestFrame()
{
setTitle("ExceptTest");
ExceptTestPanel panel = new ExceptTestPanel();
add(panel);
pack();
}
}
/**
* A panel with radio buttons for running code snippets and studying their exception behavior
*/
class ExceptTestPanel extends Box
{
public ExceptTestPanel()
{
super(BoxLayout.Y_AXIS);
group = new ButtonGroup();
// add radio buttons for code snippets
addRadioButton("Integer divide by zero", new ActionListener()
{
public void actionPerformed(ActionEvent event)
{
a[1] = 1 / (a.length - a.length);
}
});
addRadioButton("Floating point divide by zero", new ActionListener()
{
public void actionPerformed(ActionEvent event)
{
a[1] = a[2] / (a[3] - a[3]);
}
});
addRadioButton("Array bounds", new ActionListener()
{
public void actionPerformed(ActionEvent event)
{
a[1] = a[10];
}
});
addRadioButton("Bad cast", new ActionListener()
{
public void actionPerformed(ActionEvent event)
{
a = (double[]) event.getSource();
}
});
addRadioButton("Null pointer", new ActionListener()
{
public void actionPerformed(ActionEvent event)
{
event = null;
System.out.println(event.getSource());
}
});
addRadioButton("sqrt(-1)", new ActionListener()
{
public void actionPerformed(ActionEvent event)
{
a[1] = Math.sqrt(-1);
}
});
addRadioButton("Overflow", new ActionListener()
{
public void actionPerformed(ActionEvent event)
{
a[1] = 1000 * 1000 * 1000 * 1000;
int n = (int) a[1];
}
});
addRadioButton("No such file", new ActionListener()
{
public void actionPerformed(ActionEvent event)
{
try
{
InputStream in = new FileInputStream("woozle.txt");
}
catch (IOException e)
{
textField.setText(e.toString());
}
}
});
addRadioButton("Throw unknown", new ActionListener()
{
public void actionPerformed(ActionEvent event)
{
throw new UnknownError();
}
});
// add the text field for exception display
textField = new JTextField(30);
add(textField);
}
/**
* Adds a radio button with a given listener to the panel. Traps any exceptions in the
* actionPerformed method of the listener.
* @param s the label of the radio button
* @param listener the action listener for the radio button
*/
private void addRadioButton(String s, ActionListener listener)
{
JRadioButton button = new JRadioButton(s, false)
{
// the button calls this method to fire an
// action event. We override it to trap exceptions
protected void fireActionPerformed(ActionEvent event)
{
try
{
textField.setText("No exception");
super.fireActionPerformed(event);
}
catch (Exception e)
{
textField.setText(e.toString());
}
}
};
button.addActionListener(listener);
add(button);
group.add(button);
}
private ButtonGroup group;
private JTextField textField;
private double[] a = new double[10];
}
Related examples in the same category