J2ME Data Management: Record Management System
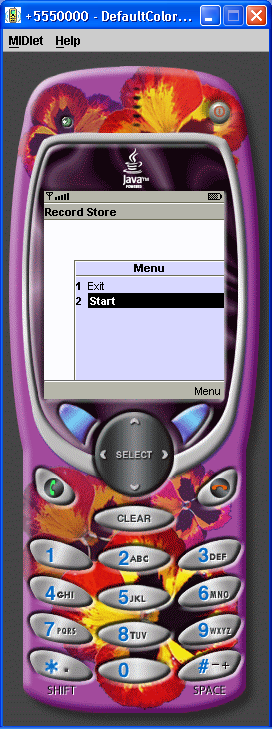
/*
J2ME: The Complete Reference
James Keogh
Publisher: McGraw-Hill
ISBN 0072227109
*/
//jad file (please verify the jar size)
/*
MIDlet-Name: RecordStoreExample
MIDlet-Version: 1.0
MIDlet-Vendor: MyCompany
MIDlet-Jar-URL: RecordStoreExample.jar
MIDlet-1: RecordStoreExample, , RecordStoreExample
MicroEdition-Configuration: CLDC-1.0
MicroEdition-Profile: MIDP-1.0
MIDlet-JAR-SIZE: 100
*/
import javax.microedition.rms.*;
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
import java.io.*;
public class RecordStoreExample
extends MIDlet implements CommandListener
{
private Display display;
private Alert alert;
private Form form;
private Command exit;
private Command start;
private RecordStore recordstore = null;
private RecordEnumeration recordenumeration = null;
public RecordStoreExample ()
{
display = Display.getDisplay(this);
exit = new Command("Exit", Command.SCREEN, 1);
start = new Command("Start", Command.SCREEN, 1);
form = new Form("Record Store");
form.addCommand(exit);
form.addCommand(start);
form.setCommandListener(this);
}
public void startApp()
{
display.setCurrent(form);
}
public void pauseApp()
{
}
public void destroyApp( boolean unconditional )
{
}
public void commandAction(Command command, Displayable displayable)
{
if (command == exit)
{
destroyApp(true);
notifyDestroyed();
}
else if (command == start)
{
try
{
recordstore = RecordStore.openRecordStore("myRecordStore",
true );
}
catch (Exception error)
{
alert = new Alert("Error Creating", error.toString(),
null, AlertType.WARNING);
alert.setTimeout(Alert.FOREVER);
display.setCurrent(alert);
}
try
{
recordstore.closeRecordStore();
}
catch (Exception error)
{
alert = new Alert("Error Closing", error.toString(),
null, AlertType.WARNING);
alert.setTimeout(Alert.FOREVER);
display.setCurrent(alert);
}
if (RecordStore.listRecordStores() != null)
{
try
{
RecordStore.deleteRecordStore("myRecordStore");
}
catch (Exception error)
{
alert = new Alert("Error Removing", error.toString(),
null, AlertType.WARNING);
alert.setTimeout(Alert.FOREVER);
display.setCurrent(alert);
}
}
}
}
}
Related examples in the same category