Display a Form and TextField for searching records. Each record contains a String object.
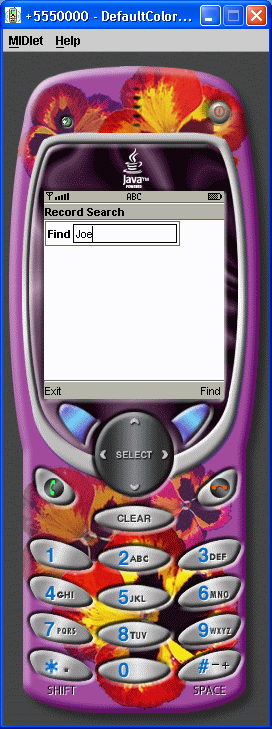
/*--------------------------------------------------
* SimpleSearch.java
*
* Display a Form and TextField for searching records
* Each record contains a String object.
*
* Uses: Enumeration, RecordFilter
*
* Example from the book: Core J2ME Technology
* Copyright John W. Muchow http://www.CoreJ2ME.com
* You may use/modify for any non-commercial purpose
*-------------------------------------------------*/
import java.io.*;
import javax.microedition.midlet.*;
import javax.microedition.rms.*;
import javax.microedition.lcdui.*;
public class SimpleSearch extends MIDlet implements CommandListener
{
private Display display; // Reference to Display object
private Form fmMain; // The main form
private StringItem siMatch; // The matching text, if any
private Command cmFind; // Command to search record store
private Command cmExit; // Command to insert items
private TextField tfFind; // Search text as requested by user
private RecordStore rs = null; // Record store
static final String REC_STORE = "db_6"; // Name of record store
public SimpleSearch()
{
display = Display.getDisplay(this);
// Define textfield, stringItem and commands
tfFind = new TextField("Find", "", 10, TextField.ANY);
siMatch = new StringItem(null, null);
cmExit = new Command("Exit", Command.EXIT, 1);
cmFind = new Command("Find", Command.SCREEN, 2);
// Create the form, add commands
fmMain = new Form("Record Search");
fmMain.addCommand(cmExit);
fmMain.addCommand(cmFind);
// Append textfield and stringItem
fmMain.append(tfFind);
fmMain.append(siMatch);
// Capture events
fmMain.setCommandListener(this);
//--------------------------------
// Open and write to record store
//--------------------------------
openRecStore(); // Create the record store
writeTestData(); // Write a series of records
}
public void destroyApp( boolean unconditional )
{
closeRecStore(); // Close record store
}
public void startApp()
{
display.setCurrent(fmMain);
}
public void pauseApp()
{
}
public void openRecStore()
{
try
{
// The second parameter indicates that the record store
// should be created if it does not exist
rs = RecordStore.openRecordStore(REC_STORE, true );
}
catch (Exception e)
{
db(e.toString());
}
}
public void closeRecStore()
{
try
{
rs.closeRecordStore();
}
catch (Exception e)
{
db(e.toString());
}
}
/*--------------------------------------------------
* Create array of data to write into record store
*-------------------------------------------------*/
public void writeTestData()
{
String[] golfClubs = {
"Wedge...good from the sand trap",
"One Wood...off the tee",
"Putter...only on the green",
"Five Iron...middle distance"};
writeRecords(golfClubs);
}
/*--------------------------------------------------
* Write to record store.
*-------------------------------------------------*/
public void writeRecords(String[] sData)
{
byte[] record;
try
{
// Only add the records once
if (rs.getNumRecords() > 0)
return;
for (int i = 0; i < sData.length; i++)
{
record = sData[i].getBytes();
rs.addRecord(record, 0, record.length);
}
}
catch (Exception e)
{
db(e.toString());
}
}
/*--------------------------------------------------
* Search using enumerator and record filter
*-------------------------------------------------*/
private void searchRecordStore()
{
try
{
// Record store is not empty
if (rs.getNumRecords() > 0)
{
// Setup the search filter with the user requested text
SearchFilter search = new SearchFilter(tfFind.getString());
RecordEnumeration re = rs.enumerateRecords(search, null, false);
// A match was found using the filter
if (re.numRecords() > 0)
// Show match in the stringItem on the form
siMatch.setText(new String(re.nextRecord()));
re.destroy(); // Free enumerator
}
}
catch (Exception e)
{
db(e.toString());
}
}
public void commandAction(Command c, Displayable s)
{
if (c == cmFind)
{
searchRecordStore();
}
else if (c == cmExit)
{
destroyApp(false);
notifyDestroyed();
}
}
/*--------------------------------------------------
* Simple message to console for debug/errors
* When used with Exceptions we should handle the
* error in a more appropriate manner.
*-------------------------------------------------*/
private void db(String str)
{
System.err.println("Msg: " + str);
}
}
/*--------------------------------------------------
* Search for text within a record
* Each record passed in contains only text (String)
*-------------------------------------------------*/
class SearchFilter implements RecordFilter
{
private String searchText = null;
public SearchFilter(String searchText)
{
// This is the text to search for
this.searchText = searchText.toLowerCase();
}
public boolean matches(byte[] candidate)
{
String str = new String(candidate).toLowerCase();
// Look for a match
if (searchText != null && str.indexOf(searchText) != -1)
return true;
else
return false;
}
}
Related examples in the same category