ImmutableImage From File
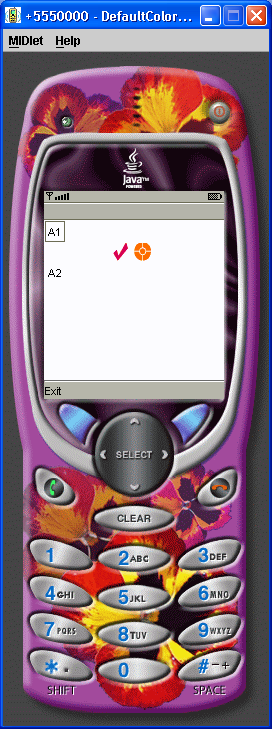
/*--------------------------------------------------
* ImmutableImageFromFile.java
*
* Example from the book: Core J2ME Technology
* Copyright John W. Muchow http://www.CoreJ2ME.com
* You may use/modify for any non-commercial purpose
*-------------------------------------------------*/
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
public class ImmutableImageFromFile extends MIDlet implements CommandListener
{
private Display display; // Reference to Display object
private Form fmMain; // The main form
private Command cmExit; // Command to exit the MIDlet
public ImmutableImageFromFile()
{
display = Display.getDisplay(this);
cmExit = new Command("Exit", Command.EXIT, 1);
fmMain = new Form("");
fmMain.addCommand(cmExit);
fmMain.setCommandListener(this);
try
{
// Read the appropriate image based on color support
Image im = Image.createImage((display.isColor()) ?
"/image_color.png":"/image_bw.png");
// Code Block A
fmMain.append("A1");
fmMain.append(new ImageItem(null, im, ImageItem.LAYOUT_NEWLINE_BEFORE |
ImageItem.LAYOUT_CENTER | ImageItem.LAYOUT_NEWLINE_AFTER, null));
fmMain.append("A2");
// Code Block B
// fmMain.append("B1");
// fmMain.append(new ImageItem(null, im,ImageItem.LAYOUT_NEWLINE_BEFORE |
// ImageItem.LAYOUT_LEFT |ImageItem.LAYOUT_NEWLINE_AFTER, null));
// fmMain.append("B2");
// Code Block C
// fmMain.append("C1");
// fmMain.append(new ImageItem(null, im,ImageItem.LAYOUT_NEWLINE_BEFORE |
// ImageItem.LAYOUT_RIGHT |ImageItem.LAYOUT_NEWLINE_AFTER, null));
// fmMain.append("C2");
// Code Block D
// fmMain.append("D1");
// fmMain.append(im);
// fmMain.append("D2");
// System.out.println("Layout Directives:" + ((ImageItem)fmMain.get(1)).getLayout());
display.setCurrent(fmMain);
}
catch (java.io.IOException e)
{
System.err.println("Unable to locate or read .png file");
}
}
public void startApp()
{
display.setCurrent(fmMain);
}
public void pauseApp()
{
}
public void destroyApp(boolean unconditional)
{
}
public void commandAction(Command c, Displayable s)
{
if (c == cmExit)
{
destroyApp(false);
notifyDestroyed();
}
}
}
Related examples in the same category