This program demonstrates collating strings under various locales.
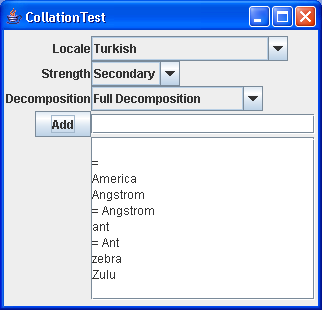
/*
This program is a part of the companion code for Core Java 8th ed.
(http://horstmann.com/corejava)
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
import java.awt.EventQueue;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Insets;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.text.Collator;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.TreeMap;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.JTextField;
/**
* This program demonstrates collating strings under various locales.
*
* @version 1.13 2007-07-25
* @author Cay Horstmann
*/
public class CollationTest {
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
JFrame frame = new CollationFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
});
}
}
/**
* This frame contains combo boxes to pick a locale, collation strength and
* decomposition rules, a text field and button to add new strings, and a text
* area to list the collated strings.
*/
class CollationFrame extends JFrame {
public CollationFrame() {
setTitle("CollationTest");
setLayout(new GridBagLayout());
add(new JLabel("Locale"), new GBC(0, 0).setAnchor(GBC.EAST));
add(new JLabel("Strength"), new GBC(0, 1).setAnchor(GBC.EAST));
add(new JLabel("Decomposition"), new GBC(0, 2).setAnchor(GBC.EAST));
add(addButton, new GBC(0, 3).setAnchor(GBC.EAST));
add(localeCombo, new GBC(1, 0).setAnchor(GBC.WEST));
add(strengthCombo, new GBC(1, 1).setAnchor(GBC.WEST));
add(decompositionCombo, new GBC(1, 2).setAnchor(GBC.WEST));
add(newWord, new GBC(1, 3).setFill(GBC.HORIZONTAL));
add(new JScrollPane(sortedWords), new GBC(0, 4, 2, 1).setFill(GBC.BOTH));
locales = (Locale[]) Collator.getAvailableLocales().clone();
Arrays.sort(locales, new Comparator<Locale>() {
private Collator collator = Collator.getInstance(Locale.getDefault());
public int compare(Locale l1, Locale l2) {
return collator.compare(l1.getDisplayName(), l2.getDisplayName());
}
});
for (Locale loc : locales)
localeCombo.addItem(loc.getDisplayName());
localeCombo.setSelectedItem(Locale.getDefault().getDisplayName());
strings.add("America");
strings.add("able");
strings.add("Zulu");
strings.add("zebra");
strings.add("\u00C5ngstr\u00F6m");
strings.add("A\u030angstro\u0308m");
strings.add("Angstrom");
strings.add("Able");
strings.add("office");
strings.add("o\uFB03ce");
strings.add("Java\u2122");
strings.add("JavaTM");
updateDisplay();
addButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent event) {
strings.add(newWord.getText());
updateDisplay();
}
});
ActionListener listener = new ActionListener() {
public void actionPerformed(ActionEvent event) {
updateDisplay();
}
};
localeCombo.addActionListener(listener);
strengthCombo.addActionListener(listener);
decompositionCombo.addActionListener(listener);
pack();
}
/**
* Updates the display and collates the strings according to the user
* settings.
*/
public void updateDisplay() {
Locale currentLocale = locales[localeCombo.getSelectedIndex()];
localeCombo.setLocale(currentLocale);
currentCollator = Collator.getInstance(currentLocale);
currentCollator.setStrength(strengthCombo.getValue());
currentCollator.setDecomposition(decompositionCombo.getValue());
Collections.sort(strings, currentCollator);
sortedWords.setText("");
for (int i = 0; i < strings.size(); i++) {
String s = strings.get(i);
if (i > 0 && currentCollator.compare(s, strings.get(i - 1)) == 0)
sortedWords.append("= ");
sortedWords.append(s + "\n");
}
pack();
}
private List<String> strings = new ArrayList<String>();
private Collator currentCollator;
private Locale[] locales;
private JComboBox localeCombo = new JComboBox();
private EnumCombo strengthCombo = new EnumCombo(Collator.class, new String[] { "Primary",
"Secondary", "Tertiary", "Identical" });
private EnumCombo decompositionCombo = new EnumCombo(Collator.class, new String[] {
"Canonical Decomposition", "Full Decomposition", "No Decomposition" });
private JTextField newWord = new JTextField(20);
private JTextArea sortedWords = new JTextArea(20, 20);
private JButton addButton = new JButton("Add");
}
/*
* This program is a part of the companion code for Core Java 8th ed.
* (http://horstmann.com/corejava)
*
* This program is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* this program. If not, see <http://www.gnu.org/licenses/>.
*/
/*
* GBC - A convenience class to tame the GridBagLayout
*
* Copyright (C) 2002 Cay S. Horstmann (http://horstmann.com)
*
* This program is free software; you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation; either version 2 of the License, or (at your option) any later
* version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* this program; if not, write to the Free Software Foundation, Inc., 59 Temple
* Place, Suite 330, Boston, MA 02111-1307 USA
*/
/**
* This class simplifies the use of the GridBagConstraints class.
*/
class GBC extends GridBagConstraints {
/**
* Constructs a GBC with a given gridx and gridy position and all other grid
* bag constraint values set to the default.
*
* @param gridx
* the gridx position
* @param gridy
* the gridy position
*/
public GBC(int gridx, int gridy) {
this.gridx = gridx;
this.gridy = gridy;
}
/**
* Constructs a GBC with given gridx, gridy, gridwidth, gridheight and all
* other grid bag constraint values set to the default.
*
* @param gridx
* the gridx position
* @param gridy
* the gridy position
* @param gridwidth
* the cell span in x-direction
* @param gridheight
* the cell span in y-direction
*/
public GBC(int gridx, int gridy, int gridwidth, int gridheight) {
this.gridx = gridx;
this.gridy = gridy;
this.gridwidth = gridwidth;
this.gridheight = gridheight;
}
/**
* Sets the anchor.
*
* @param anchor
* the anchor value
* @return this object for further modification
*/
public GBC setAnchor(int anchor) {
this.anchor = anchor;
return this;
}
/**
* Sets the fill direction.
*
* @param fill
* the fill direction
* @return this object for further modification
*/
public GBC setFill(int fill) {
this.fill = fill;
return this;
}
/**
* Sets the cell weights.
*
* @param weightx
* the cell weight in x-direction
* @param weighty
* the cell weight in y-direction
* @return this object for further modification
*/
public GBC setWeight(double weightx, double weighty) {
this.weightx = weightx;
this.weighty = weighty;
return this;
}
/**
* Sets the insets of this cell.
*
* @param distance
* the spacing to use in all directions
* @return this object for further modification
*/
public GBC setInsets(int distance) {
this.insets = new Insets(distance, distance, distance, distance);
return this;
}
/**
* Sets the insets of this cell.
*
* @param top
* the spacing to use on top
* @param left
* the spacing to use to the left
* @param bottom
* the spacing to use on the bottom
* @param right
* the spacing to use to the right
* @return this object for further modification
*/
public GBC setInsets(int top, int left, int bottom, int right) {
this.insets = new Insets(top, left, bottom, right);
return this;
}
/**
* Sets the internal padding
*
* @param ipadx
* the internal padding in x-direction
* @param ipady
* the internal padding in y-direction
* @return this object for further modification
*/
public GBC setIpad(int ipadx, int ipady) {
this.ipadx = ipadx;
this.ipady = ipady;
return this;
}
}
/*
* This program is a part of the companion code for Core Java 8th ed.
* (http://horstmann.com/corejava)
*
* This program is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* this program. If not, see <http://www.gnu.org/licenses/>.
*/
/**
* A combo box that lets users choose from among static field values whose names
* are given in the constructor.
*
* @version 1.13 2007-07-25
* @author Cay Horstmann
*/
class EnumCombo extends JComboBox {
/**
* Constructs an EnumCombo.
*
* @param cl
* a class
* @param labels
* an array of static field names of cl
*/
public EnumCombo(Class<?> cl, String[] labels) {
for (String label : labels) {
String name = label.toUpperCase().replace(' ', '_');
int value = 0;
try {
java.lang.reflect.Field f = cl.getField(name);
value = f.getInt(cl);
} catch (Exception e) {
label = "(" + label + ")";
}
table.put(label, value);
addItem(label);
}
setSelectedItem(labels[0]);
}
/**
* Returns the value of the field that the user selected.
*
* @return the static field value
*/
public int getValue() {
return table.get(getSelectedItem());
}
private Map<String, Integer> table = new TreeMap<String, Integer>();
}
Related examples in the same category