1. | Set, HashSet and TreeSet | | |
2. | Things you can do with Sets | |  |
3. | Set operations: union, intersection, difference, symmetric difference, is subset, is superset | | |
4. | Set implementation that use == instead of equals() | | |
5. | Set that compares object by identity rather than equality | | |
6. | Set union and intersection | | |
7. | Set with values iterated in insertion order. | | |
8. | Putting your own type in a Set | | 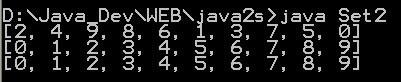 |
9. | Use set | |  |
10. | Another Set demo | | |
11. | Set subtraction | |  |
12. | Working with HashSet and TreeSet | | 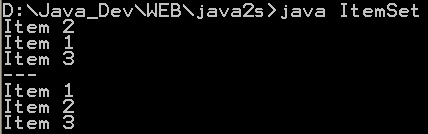 |
13. | TreeSet Demo | | 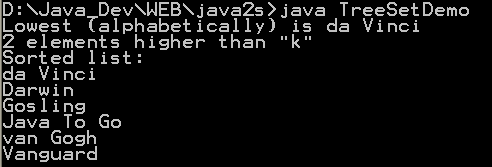 |
14. | Show the union and intersection of two sets | | |
15. | Demonstrate the Set interface | | |
16. | Array Set extends AbstractSet | | 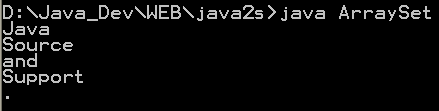 |
17. | Sync Test | | |
18. | Set Copy | | |
19. | Tail | | |
20. | What you can do with a TreeSet | | 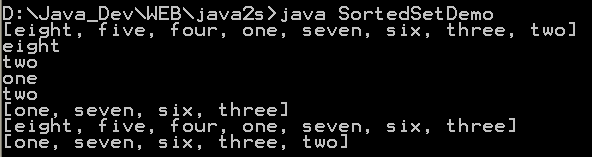 |
21. | Remove all elements from a set | | |
22. | Copy all the elements from set2 to set1 (set1 += set2), set1 becomes the union of set1 and set2 | | |
23. | Remove all the elements in set1 from set2 (set1 -= set2), set1 becomes the asymmetric difference of set1 and set2 | | |
24. | Get the intersection of set1 and set2, set1 becomes the intersection of set1 and set2 | | |
25. | Extend AbstractSet to Create Simple Set | | |
26. | Int Set | | |
27. | One Item Set | | |
28. | Small sets whose elements are known to be unique by construction | | |
29. | List Set implements Set | | |
30. | Converts a char array to a Set | | |
31. | Converts a string to a Set | | |
32. | Implements the Set interface, backed by a ConcurrentHashMap instance | | |
33. | An IdentitySet that uses reference-equality instead of object-equality | | |
34. | An implementation of the java.util.Stack based on an ArrayList instead of a Vector, so it is not synchronized to protect against multi-threaded access. | | |
35. | A thin wrapper around a List transforming it into a modifiable Set. | | |
36. | A thread-safe Set that manages canonical objects | | |
37. | This program uses a set to print all unique words in System.in | | |
38. | Indexed Set | | |
39. | An ObjectToSet provides a java.util.Map from arbitrary objects to objects of class java.util.Set. | | |
40. | Sorted Multi Set | | |
41. | Fixed Size Sorted Set | | |
42. | Set operations | | |
43. | A NumberedSet is a generic container of Objects where each element is identified by an integer id. | | |
44. | Set which counts the number of times a values are added to it. | | |
45. | Set which counts the number of times a values are added to it and assigns them a unique positive index. | | |
46. | Indexed Set | | |
47. | A set acts like array. | | |
48. | Implements a Bloom filter. Which, as you may not know, is a space-efficient structure for storing a set. | | |
49. | Implementation of disjoint-set data structure | | |
50. | Call it an unordered list or a multiset, this collection is defined by oxymorons | | |