Show the Location2Location class in action
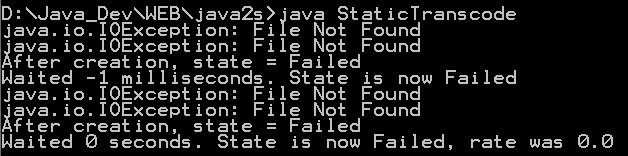
/*
Java Media APIs: Cross-Platform Imaging, Media and Visualization
Alejandro Terrazas
Sams, Published November 2002,
ISBN 0672320940
*/
import javax.media.*;
import javax.media.protocol.*;
import javax.media.format.*;
/*******************************************************************************
* Simple example to show the Location2Location class in action. The
* Location2Location class transfer media from one location to another
* performing any requested tanscoding (format changes) at the same time.
*
* The class is used twice. Once to transform a short wave audio file of an
* electric guitar (guitar.wav) into MP3 format. The second example converts of
* Quicktime version of the example video from chapter 7, encoded with the Indeo
* 5.o codec and GSM audio into an AVI version with Cinepak codec for the video
* and linear encoding for the audio.
******************************************************************************/
public class StaticTranscode {
public static void main(String[] args) {
String src;
String dest;
Format[] formats;
ContentDescriptor container;
int waited;
Location2Location dupe;
/////////////////////////////////////////////////////////////////
// Transcode a wave audio file into an MP3 file, transferring it
// to a new location (dest) at the same time.
////////////////////////////////////////////////////////////////
src = "file://d:\\jmf\\book\\media\\guitar.wav";
dest = "file://d:\\jmf\\book\\media\\guitar.mp3";
formats = new Format[1];
formats[0] = new AudioFormat(AudioFormat.MPEGLAYER3);
container = new FileTypeDescriptor(FileTypeDescriptor.MPEG_AUDIO);
dupe = new Location2Location(src, dest, formats, container);
System.out.println("After creation, state = " + dupe.getStateName());
waited = dupe.transfer(10000);
System.out.println("Waited " + waited + " milliseconds. State is now "
+ dupe.getStateName());
///////////////////////////////////////////////////////////////////
// Transcode a Quicktime version of a movie into an AVI version.
// The video codec is altered from Indeo5.0 to Cinepak,the audio
// track is transcoded from GSM to linear, and is result is saved
// as a file "qaz.avi".
//////////////////////////////////////////////////////////////////
src = "file://d:\\jmf\\book\\media\\videoexample\\iv50_320x240.mov";
dest = "file://d:\\jmf\\book\\media\\qaz.avi";
formats = new Format[2];
formats[0] = new VideoFormat(VideoFormat.CINEPAK);
formats[1] = new AudioFormat(AudioFormat.LINEAR);
container = new FileTypeDescriptor(FileTypeDescriptor.MSVIDEO);
dupe = new Location2Location(src, dest, formats, container, 5.0f);
System.out.println("After creation, state = " + dupe.getStateName());
waited = dupe.transfer(Location2Location.INDEFINITE);
int state = dupe.getState();
System.out.println("Waited " + (waited / 1000)
+ " seconds. State is now " + dupe.getStateName()
+ ", rate was " + dupe.getRate());
System.exit(0);
}
}
Related examples in the same category