Java Media: Find Components
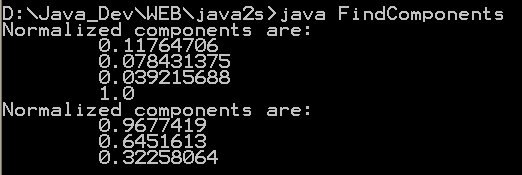
/*
Java Media APIs: Cross-Platform Imaging, Media and Visualization
Alejandro Terrazas
Sams, Published November 2002,
ISBN 0672320940
*/
import java.awt.image.DirectColorModel;
public class FindComponents {
DirectColorModel dcm32;
DirectColorModel dcm16;
int[] components;
float[] componentsf;
int value32;
short value16;
int red8, green8, blue8, alpha8;
short red5, green5, blue5;
/**
* FindComponents.java -- prints out normalized color components for two
* different
*/
public FindComponents() {
red8 = red5 = 30;
green8 = green5 = 20;
blue8 = blue5 = 10;
alpha8 = 255;
dcm32 = new DirectColorModel(32, 0x00ff0000, 0x0000ff00, 0x000000ff,
0xff000000);
value32 = (alpha8 << 24) + (red8 << 16) + (green8 << 8) + blue8;
components = dcm32.getComponents(value32, null, 0);
componentsf = dcm32.getNormalizedComponents(components, 0, null, 0);
System.out.println("Normalized components are: ");
for (int i = 0; i < componentsf.length; i++)
System.out.println("\t" + componentsf[i]);
dcm16 = new DirectColorModel(16, 0x7c00, 0x3e0, 0x1f);
value16 = (short) ((red5 << 10) + (green5 << 5) + blue5);
components = dcm16.getComponents(value16, null, 0);
componentsf = dcm16.getNormalizedComponents(components, 0, null, 0);
System.out.println("Normalized components are: ");
for (int i = 0; i < componentsf.length; i++)
System.out.println("\t" + componentsf[i]);
}
public static void main(String[] args) {
new FindComponents();
}
}
Related examples in the same category