List Binding
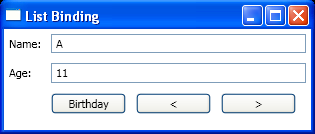
<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:WpfApplication1"
Title="List Binding" Height="135" Width="315">
<Window.Resources>
<local:People x:Key="Family">
<local:Person Name="A" Age="11" />
<local:Person Name="B" Age="27" />
<local:Person Name="C" Age="38" />
</local:People>
<local:AgeToForegroundConverter x:Key="ageConverter" />
</Window.Resources>
<Grid DataContext="{StaticResource Family}">
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto" />
<ColumnDefinition Width="*" />
</Grid.ColumnDefinitions>
<TextBlock Grid.Row="0" Grid.Column="0" Margin="5" VerticalAlignment="Center">Name:</TextBlock>
<TextBox Grid.Row="0" Grid.Column="1" Margin="5" Text="{Binding Path=Name}" />
<TextBlock Grid.Row="1" Grid.Column="0" Margin="5" VerticalAlignment="Center">Age:</TextBlock>
<TextBox Grid.Row="1" Grid.Column="1" Margin="5" Text="{Binding Path=Age}"
Foreground="{Binding Path=Age, Converter={StaticResource ageConverter}}" />
<StackPanel Grid.Row="2" Grid.Column="1" Orientation="Horizontal">
<Button Name="birthdayButton" Width="75" Margin="5">Birthday</Button>
<Button Name="backButton" Width="75" Margin="5"><</Button>
<Button Name="forwardButton" Width="75" Margin="5">></Button>
</StackPanel>
</Grid>
</Window>
//File:Window.xaml.cs
using System;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
using System.ComponentModel;
using System.Collections.Generic;
using System.Diagnostics;
namespace WpfApplication1 {
public class Person : INotifyPropertyChanged {
public event PropertyChangedEventHandler PropertyChanged;
protected void Notify(string propName) {
if( this.PropertyChanged != null ) {
PropertyChanged(this, new PropertyChangedEventArgs(propName));
}
}
string name;
public string Name {
get { return this.name; }
set {
if( this.name == value ) { return; }
this.name = value;
Notify("Name");
}
}
int age;
public int Age {
get { return this.age; }
set {
if( this.age == value ) { return; }
this.age = value;
Notify("Age");
}
}
public Person() { }
public Person(string name, int age) {
this.name = name;
this.age = age;
}
}
class People : List<Person> { }
public partial class Window1 : Window {
public Window1() {
InitializeComponent();
this.birthdayButton.Click += birthdayButton_Click;
this.backButton.Click += backButton_Click;
this.forwardButton.Click += forwardButton_Click;
}
ICollectionView GetFamilyView() {
People people = (People)this.FindResource("Family");
return CollectionViewSource.GetDefaultView(people);
}
void birthdayButton_Click(object sender, RoutedEventArgs e) {
ICollectionView view = GetFamilyView();
Person person = (Person)view.CurrentItem;
++person.Age;
System.Console.WriteLine(person.Name);
System.Console.WriteLine(person.Age);
}
void backButton_Click(object sender, RoutedEventArgs e) {
ICollectionView view = GetFamilyView();
view.MoveCurrentToPrevious();
if( view.IsCurrentBeforeFirst ) {
view.MoveCurrentToFirst();
}
}
void forwardButton_Click(object sender, RoutedEventArgs e) {
ICollectionView view = GetFamilyView();
view.MoveCurrentToNext();
if( view.IsCurrentAfterLast ) {
view.MoveCurrentToLast();
}
}
}
public class AgeToForegroundConverter : IValueConverter {
public object Convert(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture) {
Debug.Assert(targetType == typeof(Brush));
int age = int.Parse(value.ToString());
return (age > 25 ? Brushes.Red : Brushes.Black);
}
public object ConvertBack(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture) {
throw new NotImplementedException();
}
}
}
Related examples in the same category