Dotted Path
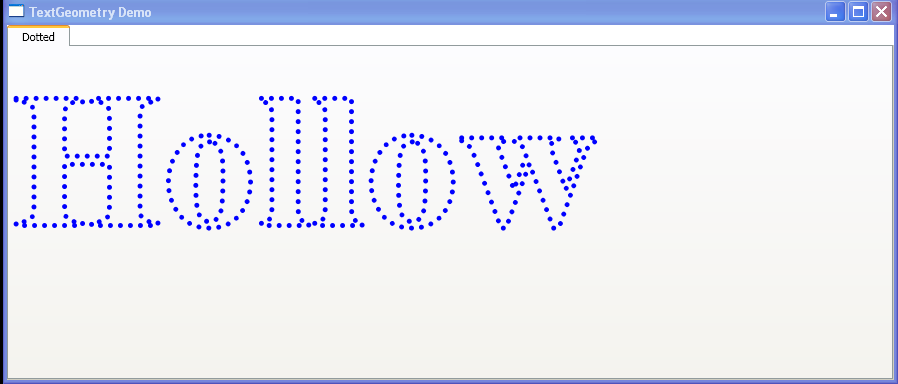
<Window xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:src="clr-namespace:MyNameSpace.TextGeometryDemo"
Title="TextGeometry Demo">
<Window.Resources>
<src:TextGeometry x:Key="txtHollow" Text="Hollow"
FontFamily="Times New Roman"
FontSize="192" FontWeight="Bold" />
<src:TextGeometry x:Key="txtShadow" Text="Shadow"
FontFamily="Times New Roman"
FontSize="192" FontWeight="Bold" />
</Window.Resources>
<TabControl>
<TabItem Header="Dotted">
<Path Stroke="Blue" StrokeThickness="5"
StrokeDashArray="{Binding Source={x:Static DashStyles.Dot},Path=Dashes}"
StrokeDashCap="Round"
Data="{Binding Source={StaticResource txtHollow},Path=Geometry}" />
</TabItem>
</TabControl>
</Window>
//File:Window.xaml.cs
using System;
using System.Globalization;
using System.Windows;
using System.Windows.Media;
namespace MyNameSpace.TextGeometryDemo
{
public class TextGeometry
{
string txt = "";
FontFamily fntfam = new FontFamily();
FontStyle fntstyle = FontStyles.Normal;
FontWeight fntwt = FontWeights.Normal;
FontStretch fntstr = FontStretches.Normal;
double emsize = 24;
Point ptOrigin = new Point(0, 0);
public string Text
{
set { txt = value; }
get { return txt; }
}
public FontFamily FontFamily
{
set { fntfam = value; }
get { return fntfam; }
}
public FontStyle FontStyle
{
set { fntstyle = value; }
get { return fntstyle; }
}
public FontWeight FontWeight
{
set { fntwt = value; }
get { return fntwt; }
}
public FontStretch FontStretch
{
set { fntstr = value; }
get { return fntstr; }
}
public double FontSize
{
set { emsize = value; }
get { return emsize; }
}
public Point Origin
{
set { ptOrigin = value; }
get { return ptOrigin; }
}
public Geometry Geometry
{
get
{
FormattedText formtxt = new FormattedText(Text, CultureInfo.CurrentCulture,
FlowDirection.LeftToRight,
new Typeface(FontFamily, FontStyle,FontWeight, FontStretch),
FontSize, Brushes.Black);
return formtxt.BuildGeometry(Origin);
}
}
public PathGeometry PathGeometry
{
get
{
return PathGeometry.CreateFromGeometry(Geometry);
}
}
}
}
Related examples in the same category
1. | StrokeDashArray 4,2 / StrokeDashOffset: 0 | | 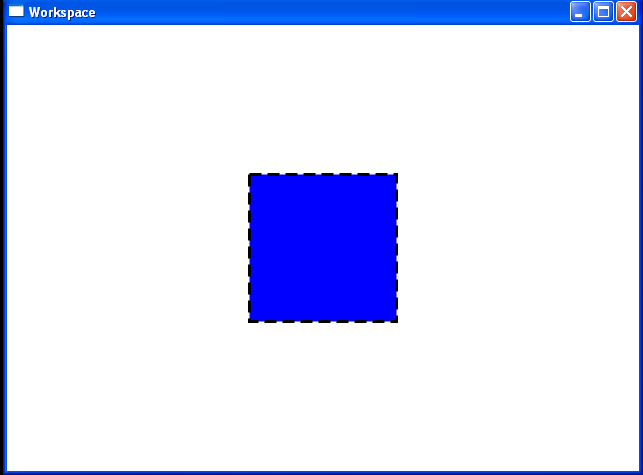 |
2. | StrokeDashArray>: 4,2 /StrokeDashOffset: 1 | | 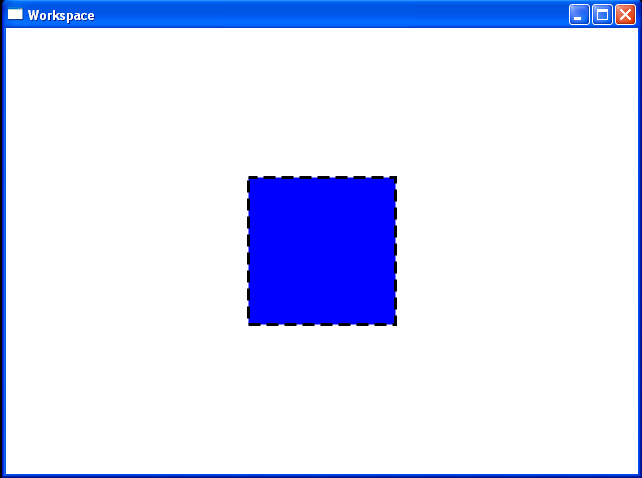 |
3. | StrokeDashArray: 4,2 /StrokeDashOffset: 2 | | 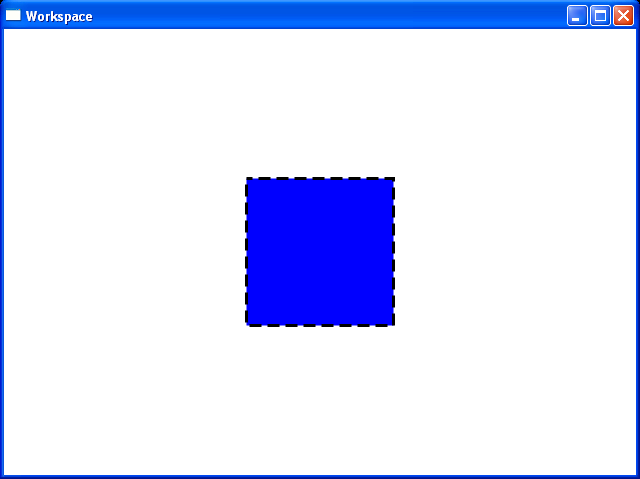 |
4. | StrokeDashArray: 4,1,4,3 /StrokeDashOffset: 1 | | 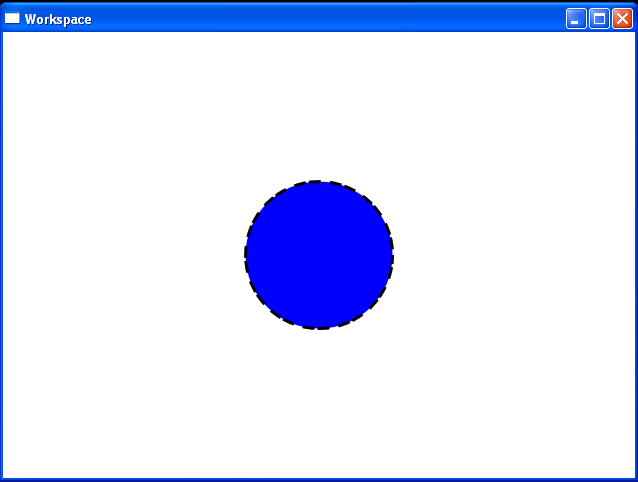 |
5. | StrokeDashArray: 1,4,1,2 / StrokeDashOffset: 1 | | 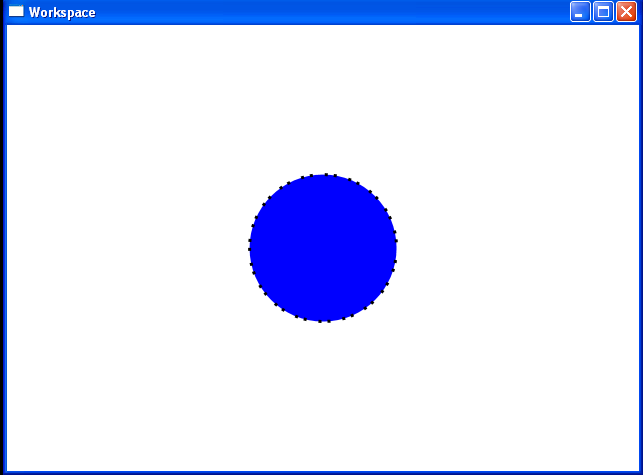 |
6. | StrokeDashArray: 1 / StrokeDashOffset: 1 | | 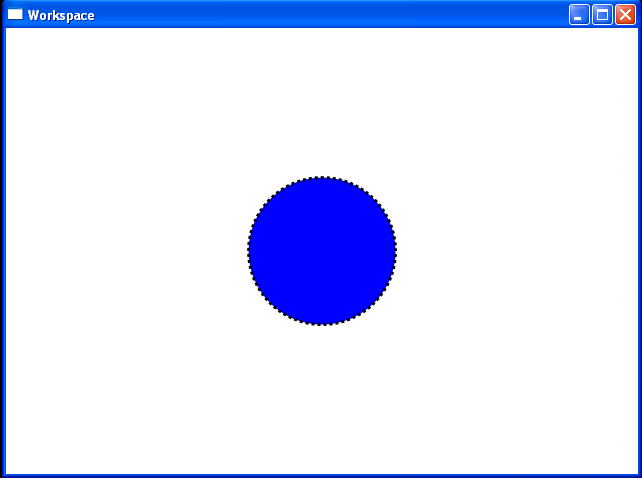 |
7. | PenLineJoin.Bevel | | 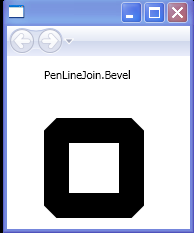 |
8. | PenLineJoin.Round | | 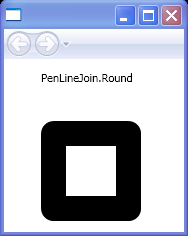 |
9. | PenLineJoin.Miter | | 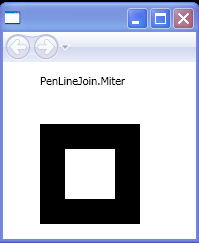 |
10. | PenLineCap.Flat | | 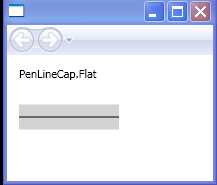 |
11. | PenLineCap.Square | | 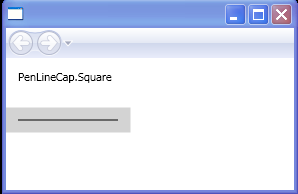 |
12. | PenLineCap.Round | | 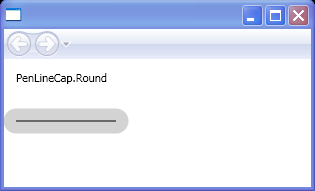 |
13. | PenLineCap.Triangle | | 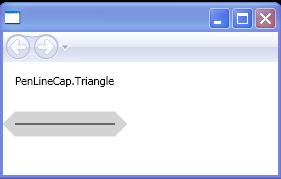 |
14. | StrokeStartLineCap=Round, StrokeEndLineCap=Round | | 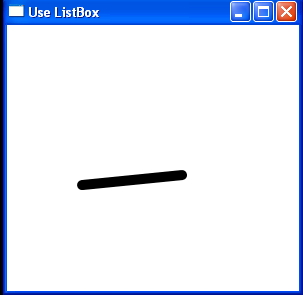 |