Demonstrates adding multiple methods to a delegate
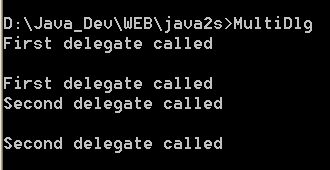
/*
C# Programming Tips & Techniques
by Charles Wright, Kris Jamsa
Publisher: Osborne/McGraw-Hill (December 28, 2001)
ISBN: 0072193794
*/
// MultiDlg.cs -- Demonstrates adding multiple methods to a delegate.
//
// Compile this program with the following command line
// C:>csc MultiDlg.cs
using System;
namespace nsDelegates
{
public class MultiDlg
{
public delegate void MultiMethod ();
static public void Main ()
{
MultiMethod dlg;
// Assign the first method to the delegate.
dlg = new MultiMethod (FirstDelegate);
// Call it to show the first method is being called
dlg ();
// Add a second method to the delegate.
dlg += new MultiMethod (SecondDelegate);
// Call it to show both methods execute.
Console.WriteLine ();
dlg ();
// Remove the first method from the delegate.
dlg -= new MultiMethod (FirstDelegate);
// Call it to show that only the second method executes
Console.WriteLine ();
dlg ();
}
static public void FirstDelegate()
{
Console.WriteLine ("First delegate called");
}
static public void SecondDelegate()
{
Console.WriteLine ("Second delegate called");
}
}
}
Related examples in the same category