This program averages a list of numbers entered by the user
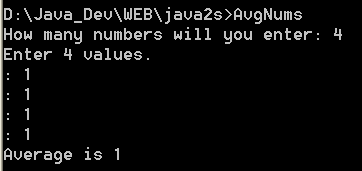
/*
C#: The Complete Reference
by Herbert Schildt
Publisher: Osborne/McGraw-Hill (March 8, 2002)
ISBN: 0072134852
*/
// This program averages a list of numbers entered by the user.
using System;
using System.IO;
public class AvgNums {
public static void Main() {
string str;
int n;
double sum = 0.0;
double avg, t;
Console.Write("How many numbers will you enter: ");
str = Console.ReadLine();
try {
n = Int32.Parse(str);
}
catch(FormatException exc) {
Console.WriteLine(exc.Message);
n = 0;
}
catch(OverflowException exc) {
Console.WriteLine(exc.Message);
n = 0;
}
Console.WriteLine("Enter " + n + " values.");
for(int i=0; i < n ; i++) {
Console.Write(": ");
str = Console.ReadLine();
try {
t = Double.Parse(str);
} catch(FormatException exc) {
Console.WriteLine(exc.Message);
t = 0.0;
}
catch(OverflowException exc) {
Console.WriteLine(exc.Message);
t = 0;
}
sum += t;
}
avg = sum / n;
Console.WriteLine("Average is " + avg);
}
}
Related examples in the same category