Read a line from console
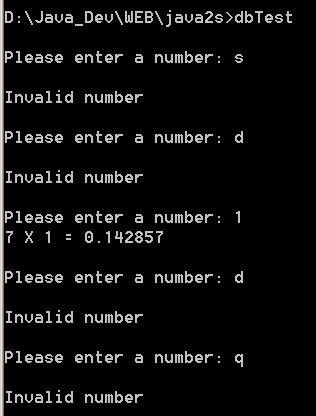
/*
C# Programming Tips & Techniques
by Charles Wright, Kris Jamsa
Publisher: Osborne/McGraw-Hill (December 28, 2001)
ISBN: 0072193794
*/
// dbTest.cs -- Sample program to be used with cordbg.exe
//
// Compile this program with the following command line:
// C:>csc /debug:full dbTest.cs
//
using System;
namespace nsDebug
{
public class dbTest
{
static public void Main ()
{
double x = 7.0;
while (true)
{
Console.Write ("\r\nPlease enter a number: ");
string str = Console.ReadLine ();
if (str.Length == 0)
break;
double val = 0;
try
{
val = Convert.ToDouble (str);
}
catch (Exception)
{
Console.WriteLine ("\r\nInvalid number");
continue;
}
Console.WriteLine (x + " X " + val + " = {0,0:F6}", val / x);
}
}
}
}
Related examples in the same category