Set Text Output To Event Log
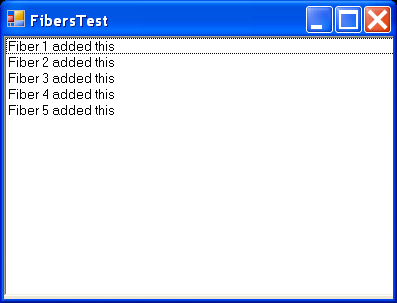
/*
Professional Windows GUI Programming Using C#
by Jay Glynn, Csaba Torok, Richard Conway, Wahid Choudhury,
Zach Greenvoss, Shripad Kulkarni, Neil Whitlow
Publisher: Peer Information
ISBN: 1861007663
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Runtime.InteropServices;
using System.Threading;
using System.Diagnostics;
namespace FibersTest
{
/// <summary>
/// Summary description for Form1.
/// </summary>
///
public class fmrFibers : System.Windows.Forms.Form
{
private System.Windows.Forms.ListBox lstFibers;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
[DllImport("kernel32.dll")]
extern static IntPtr ConvertThreadToFiber(int fiberData);
[DllImport("kernel32.dll")]
extern static IntPtr CreateFiber(int size, System.Delegate function, int handle);
[DllImport("kernel32.dll")]
extern static IntPtr SwitchToFiber(IntPtr fiberAddress);
[DllImport("kernel32.dll")]
extern static void DeleteFiber(IntPtr fiberAddress);
[DllImport("kernel32.dll")]
extern static int GetLastError();
delegate void SetTextOutputToEventLog(int number);
public fmrFibers()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
Thread t1 = new Thread(new ThreadStart(NewThreadToFiberExecution));
t1.Start();
}
void OutputLog(int fiberNumber)
{
this.Invoke(new AddToListBox(SetText), new object[]{fiberNumber});
SwitchToFiber(obj);
}
void SetText(int message)
{
lstFibers.Items.Add("Fiber "+message.ToString()+" added this");
}
delegate void AddToListBox(int message);
System.IntPtr obj;
void NewThreadToFiberExecution()
{
try
{
SetTextOutputToEventLog stof = new SetTextOutputToEventLog(OutputLog);
obj = ConvertThreadToFiber(0);
long l1 = GetLastError();
System.IntPtr retVal1 = CreateFiber(500, stof, 1);
System.IntPtr retVal2 = CreateFiber(500, stof, 2);
System.IntPtr retVal3 = CreateFiber(500, stof, 3);
System.IntPtr retVal4 = CreateFiber(500, stof, 4);
System.IntPtr retVal5 = CreateFiber(500, stof, 5);
if(GetLastError()!=0) throw new Exception("Create Fiber failed!!");
IntPtr fiber1return = SwitchToFiber(retVal1);
IntPtr fiber2return = SwitchToFiber(retVal2);
IntPtr fiber3return = SwitchToFiber(retVal3);
IntPtr fiber4return = SwitchToFiber(retVal4);
IntPtr fiber5return = SwitchToFiber(retVal5);
if(GetLastError()!=0) throw new Exception("Create Fiber failed!!");
DeleteFiber(retVal1);
DeleteFiber(retVal2);
DeleteFiber(retVal3);
DeleteFiber(retVal4);
DeleteFiber(retVal5);
}
catch(Exception e)
{
throw e;
}
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.lstFibers = new System.Windows.Forms.ListBox();
this.SuspendLayout();
//
// lstFibers
//
this.lstFibers.Name = "lstFibers";
this.lstFibers.Size = new System.Drawing.Size(320, 212);
this.lstFibers.TabIndex = 0;
//
// fmrFibers
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(304, 213);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.lstFibers});
this.Name = "fmrFibers";
this.Text = "FibersTest";
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new fmrFibers());
}
}
}
Related examples in the same category