Shifts the given value into the range
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Xml;
namespace Microsoft.SqlServer.SpatialToolbox.KMLProcessor
{
public class Utilities
{
/// <summary>
/// This method shifts the given value into the range [-upperBorder, upperBorder]
/// </summary>
/// <param name="value">The value to be shifted</param>
/// <param name="phase">The range's upper bound</param>
/// <returns>The shifted value</returns>
public static double ShiftInRange(double value, double upperBorder)
{
upperBorder = Math.Abs(upperBorder);
if (upperBorder == 0)
throw new Exception("The range has to be greater then 0.");
double rangeSize = 2 * upperBorder;
if (value > upperBorder)
{
int k = (int)((value + upperBorder) / rangeSize);
value = value - k * rangeSize;
}
else if (value < -1 * upperBorder)
{
int k = (int)((value - upperBorder) / rangeSize);
value = value - k * rangeSize;
}
return value;
}
}
}
Related examples in the same category
1. | Compute the area of a circle | |  |
2. | the differences
between int and double | |  |
3. | Implement the Pythagorean Theorem | | 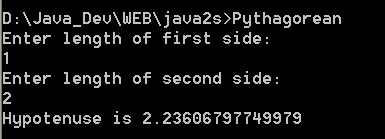 |
4. | Talking to Mars: double value calculation | |  |
5. | converts Fahrenheit to Celsius | |  |
6. | Epsilon, PositiveInfinity, NegativeInfinity, MaxValue, MinValue | | |
7. | double number format: 0:C, 0:D9, 0:E, 0:F3, 0:N, 0:X, 0:x | | |
8. | Format double value | | |
9. | Get Decimal Places | | |
10. | Automatic conversion from double to string | | |
11. | An int, a short, a float, and a double are added together giving a double result. | | |
12. | Test to see if a double is a finite number (is not NaN or Infinity). | | |
13. | Double.Epsilon Field represents the smallest positive Double value that is greater than zero. | | |
14. | Availible Double Range | | |
15. | Truncates the specified double. | | |
16. | Is Nearly Equal | | |