Availible Double Range
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Schedule.Utils
{
public class AvailibleDoubleRange
{
private double _start;
private double _end;
public double Start { get { return _start; } internal set { _start = value; } }
public double End { get { return _end; } internal set { _end = value; } }
public AvailibleDoubleRange(double start, double end)
{
_start = start;
_end = end;
AvailibleRange = new DoubleRange[] { new DoubleRange() { Start = start, End = end } };
}
public DoubleRange[] AvailibleRange { get; private set; }
public void SubtractRange(Double start, Double end)
{
List<DoubleRange> newRanges = new List<DoubleRange>(AvailibleRange);
for (int i = newRanges.Count - 1; i >= 0; i--)
{
if (start <= newRanges[i].Start && end >= newRanges[i].End)
{
// subtract range is larger then item, remove the item
newRanges.RemoveAt(i);
}
else if (start == newRanges[i].Start)
{
// if the range start is equal to the start of the other item, adjust start
newRanges[i] = new DoubleRange(end, newRanges[i].End);
}
else if (end == newRanges[i].End)
{
// if the range end is equal to the start of the other item, adjust end
newRanges[i] = new DoubleRange(newRanges[i].Start, start);
}
else if (start > newRanges[i].Start && end < newRanges[i].End)
{
// split the ranges
DoubleRange leftRange = new DoubleRange(){ Start = newRanges[i].Start, End = start};
DoubleRange rightRange = new DoubleRange() { Start = end, End = newRanges[i].End };
newRanges.RemoveAt(i);
newRanges.Add(leftRange);
newRanges.Add(rightRange);
}
}
AvailibleRange = newRanges.ToArray();
}
public void AddRange(DoubleRange range)
{
throw new NotSupportedException();
}
internal AvailibleDoubleRange Clone()
{
AvailibleDoubleRange copy = new AvailibleDoubleRange(this.Start, this.End);
copy.AvailibleRange = this.AvailibleRange.Clone() as DoubleRange[];
return copy;
}
}
public struct DoubleRange
{
private double _start;
private double _end;
public DoubleRange(double start, double end)
{
_start = start;
_end = end;
}
public double Start { get { return _start; } internal set { _start = value; } }
public double End { get { return _end; } internal set { _end = value; } }
}
}
Related examples in the same category
1. | Compute the area of a circle | |  |
2. | the differences
between int and double | |  |
3. | Implement the Pythagorean Theorem | | 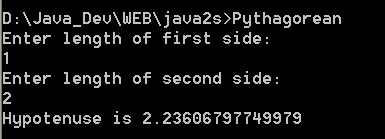 |
4. | Talking to Mars: double value calculation | |  |
5. | converts Fahrenheit to Celsius | |  |
6. | Epsilon, PositiveInfinity, NegativeInfinity, MaxValue, MinValue | | |
7. | double number format: 0:C, 0:D9, 0:E, 0:F3, 0:N, 0:X, 0:x | | |
8. | Format double value | | |
9. | Get Decimal Places | | |
10. | Automatic conversion from double to string | | |
11. | An int, a short, a float, and a double are added together giving a double result. | | |
12. | Test to see if a double is a finite number (is not NaN or Infinity). | | |
13. | Double.Epsilon Field represents the smallest positive Double value that is greater than zero. | | |
14. | Truncates the specified double. | | |
15. | Is Nearly Equal | | |
16. | Shifts the given value into the range | | |