Compare strings
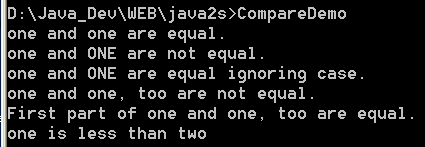
/*
C#: The Complete Reference
by Herbert Schildt
Publisher: Osborne/McGraw-Hill (March 8, 2002)
ISBN: 0072134852
*/
// Compare strings.
using System;
public class CompareDemo {
public static void Main() {
string str1 = "one";
string str2 = "one";
string str3 = "ONE";
string str4 = "two";
string str5 = "one, too";
if(String.Compare(str1, str2) == 0)
Console.WriteLine(str1 + " and " + str2 +
" are equal.");
else
Console.WriteLine(str1 + " and " + str2 +
" are not equal.");
if(String.Compare(str1, str3) == 0)
Console.WriteLine(str1 + " and " + str3 +
" are equal.");
else
Console.WriteLine(str1 + " and " + str3 +
" are not equal.");
if(String.Compare(str1, str3, true) == 0)
Console.WriteLine(str1 + " and " + str3 +
" are equal ignoring case.");
else
Console.WriteLine(str1 + " and " + str3 +
" are not equal ignoring case.");
if(String.Compare(str1, str5) == 0)
Console.WriteLine(str1 + " and " + str5 +
" are equal.");
else
Console.WriteLine(str1 + " and " + str5 +
" are not equal.");
if(String.Compare(str1, 0, str5, 0, 3) == 0)
Console.WriteLine("First part of " + str1 + " and " +
str5 + " are equal.");
else
Console.WriteLine("First part of " + str1 + " and " +
str5 + " are not equal.");
int result = String.Compare(str1, str4);
if(result < 0)
Console.WriteLine(str1 + " is less than " + str4);
else if(result > 0)
Console.WriteLine(str1 + " is greater than " + str4);
else
Console.WriteLine(str1 + " equals " + str4);
}
}
Related examples in the same category
1. | Test for equality between stings. | | |
2. | use the Equals() method and equality operator to check if two strings are equal | | |
3. | use the Compare() method to compare strings | | |
4. | Comparing a String to the Beginning or End of a Second String | | 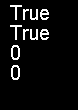 |
5. | String copy and equal | | 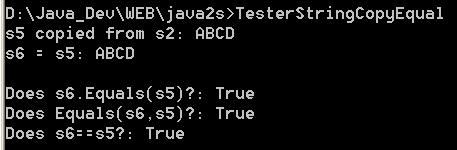 |
6. | Use String.Compare to check if a URI is a file URI | | |
7. | Sample for String.Compare(String, Int32, String, Int32, Int32, Boolean), ignore case | | |
8. | Sample for String.Compare(String, Int32, String, Int32, Int32, Boolean), Honor case | | |
9. | Check if a String contain another string by ignoring case | | |
10. | Compare two strings with StringComparison.CurrentCultureIgnoreCase | | |
11. | Checks if the string starts with special character. This does not include any special character from AllowedSpecialCharacters | | |