Checks if the string starts with special character. This does not include any special character from AllowedSpecialCharacters
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace WebOssApplications.Common
{
public static class Extensions
{
/// <summary>
/// Checks if the string starts with special character. This does not include any special character from <see cref="AllowedSpecialCharacters"/>
/// </summary>
/// <param name="value">Value to check</param>
/// <returns>True if the string starts with a special character</returns>
/// <exception cref="ArgumentNullException">Throws if value is null</exception>
/// <exception cref="ArgumentOutOfRangeException">Throws if value is empty</exception>
public static bool StartsWithSymbol(this string value)
{
if (value == null)
{
throw new ArgumentNullException("value");
}
if (string.IsNullOrEmpty(value))
{
throw new ArgumentOutOfRangeException("value");
}
return char.IsSymbol(value, 0) || char.IsPunctuation(value, 0);
}
}
}
Related examples in the same category
1. | Test for equality between stings. | | |
2. | use the Equals() method and equality operator to check if two strings are equal | | |
3. | use the Compare() method to compare strings | | |
4. | Comparing a String to the Beginning or End of a Second String | | 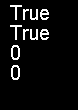 |
5. | Compare strings | | 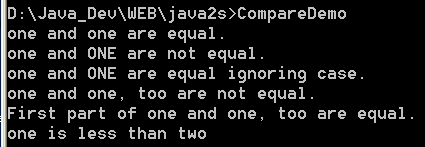 |
6. | String copy and equal | | 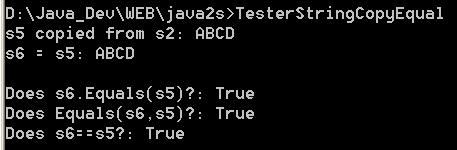 |
7. | Use String.Compare to check if a URI is a file URI | | |
8. | Sample for String.Compare(String, Int32, String, Int32, Int32, Boolean), ignore case | | |
9. | Sample for String.Compare(String, Int32, String, Int32, Int32, Boolean), Honor case | | |
10. | Check if a String contain another string by ignoring case | | |
11. | Compare two strings with StringComparison.CurrentCultureIgnoreCase | | |