Implements the stack data type using an array
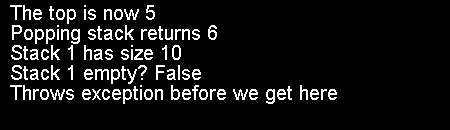
using System;
public class Stack {
private int[] data;
private int size;
private int top = -1;
public Stack() {
size = 10;
data = new int[size];
}
public Stack(int size) {
this.size = size;
data = new int[size];
}
public bool IsEmpty() {
return top == -1;
}
public bool IsFull() {
return top == size - 1;
}
public void Push(int i){
if (IsFull())
throw new ApplicationException("Stack full");
else
data[++top] = i;
}
public int Pop(){
if (IsEmpty())
throw new StackEmptyException("Stack empty");
else
return data[top--];
}
public int Top(){
if (IsEmpty())
throw new StackEmptyException("Stack empty");
else
return data[top];
}
public static void Main() {
try {
Stack stack1 = new Stack();
stack1.Push(4);
stack1.Push(5);
Console.WriteLine("The top is now {0}", stack1.Top());
stack1.Push(6);
Console.WriteLine("Popping stack returns {0}", stack1.Pop());
Console.WriteLine("Stack 1 has size {0}", stack1.size);
Console.WriteLine("Stack 1 empty? {0}", stack1.IsEmpty());
stack1.Pop();
Console.WriteLine("Throws exception before we get here");
}catch(Exception e) {
Console.WriteLine(e);
}
}
}
class StackEmptyException : ApplicationException {
public StackEmptyException(String message) : base(message) {
}
}
Related examples in the same category