Demonstrate the Stack class
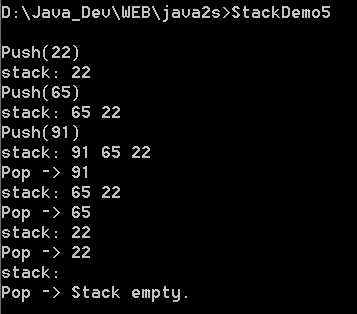
/*
C#: The Complete Reference
by Herbert Schildt
Publisher: Osborne/McGraw-Hill (March 8, 2002)
ISBN: 0072134852
*/
// Demonstrate the Stack class.
using System;
using System.Collections;
public class StackDemo5 {
static void showPush(Stack st, int a) {
st.Push(a);
Console.WriteLine("Push(" + a + ")");
Console.Write("stack: ");
foreach(int i in st)
Console.Write(i + " ");
Console.WriteLine();
}
static void showPop(Stack st) {
Console.Write("Pop -> ");
int a = (int) st.Pop();
Console.WriteLine(a);
Console.Write("stack: ");
foreach(int i in st)
Console.Write(i + " ");
Console.WriteLine();
}
public static void Main() {
Stack st = new Stack();
foreach(int i in st)
Console.Write(i + " ");
Console.WriteLine();
showPush(st, 22);
showPush(st, 65);
showPush(st, 91);
showPop(st);
showPop(st);
showPop(st);
try {
showPop(st);
} catch (InvalidOperationException) {
Console.WriteLine("Stack empty.");
}
}
}
Related examples in the same category