Illustrates how to assign default values to fields using initializers
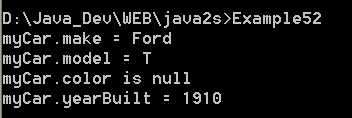
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example5_2.cs illustrates how to assign default values
to fields using initializers
*/
// declare the Car class
class Car
{
// declare the fields
public string make = "Ford";
public string model = "T";
public string color; // default value of null
public int yearBuilt = 1910;
// define the methods
public void Start()
{
System.Console.WriteLine(model + " started");
}
public void Stop()
{
System.Console.WriteLine(model + " stopped");
}
}
public class Example5_2
{
public static void Main()
{
// create a Car object
Car myCar = new Car();
// display the default values for the Car object fields
System.Console.WriteLine("myCar.make = " + myCar.make);
System.Console.WriteLine("myCar.model = " + myCar.model);
if (myCar.color == null)
{
System.Console.WriteLine("myCar.color is null");
}
System.Console.WriteLine("myCar.yearBuilt = " + myCar.yearBuilt);
}
}
Related examples in the same category