A program that uses the Building class
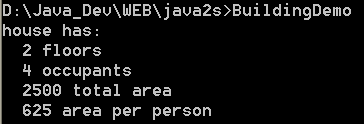
/*
C#: The Complete Reference
by Herbert Schildt
Publisher: Osborne/McGraw-Hill (March 8, 2002)
ISBN: 0072134852
*/
// A program that uses the Building class.
using System;
class Building {
public int floors; // number of floors
public int area; // total square footage of building
public int occupants; // number of occupants
}
// This class declares an object of type Building.
public class BuildingDemo {
public static void Main() {
Building house = new Building(); // create a Building object
int areaPP; // area per person
// assign values to fields in house
house.occupants = 4;
house.area = 2500;
house.floors = 2;
// compute the area per person
areaPP = house.area / house.occupants;
Console.WriteLine("house has:\n " +
house.floors + " floors\n " +
house.occupants + " occupants\n " +
house.area + " total area\n " +
areaPP + " area per person");
}
}
Related examples in the same category