Is click inside a path
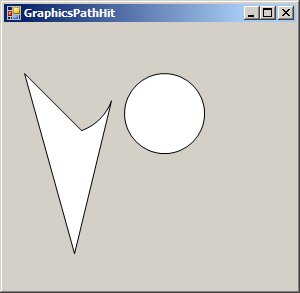
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Drawing.Drawing2D;
public class Form1 : Form
{
GraphicsPath path;
public Form1() {
InitializeComponent();
}
private void GraphicsPathHit_Paint(object sender, PaintEventArgs e)
{
e.Graphics.SmoothingMode = SmoothingMode.AntiAlias;
path = new GraphicsPath();
path.StartFigure();
path.AddArc(10, 10, 100, 100, 20, 50);
path.AddLine(20, 50, 70, 230);
path.CloseFigure();
path.AddEllipse(120, 50, 80, 80);
e.Graphics.FillPath(Brushes.White, path);
e.Graphics.DrawPath(Pens.Black, path);
}
private void GraphicsPathHit_MouseDown(object sender, MouseEventArgs e)
{
if (path.IsVisible(e.X, e.Y))
{
MessageBox.Show("You clicked inside the figure.");
}
}
private void InitializeComponent()
{
this.SuspendLayout();
//
// GraphicsPathHit
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(292, 266);
this.Name = "GraphicsPathHit";
this.Text = "GraphicsPathHit";
this.Paint += new System.Windows.Forms.PaintEventHandler(this.GraphicsPathHit_Paint);
this.MouseDown += new System.Windows.Forms.MouseEventHandler(this.GraphicsPathHit_MouseDown);
this.ResumeLayout(false);
}
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.Run(new Form1());
}
}
Related examples in the same category