Font size, name and strike out
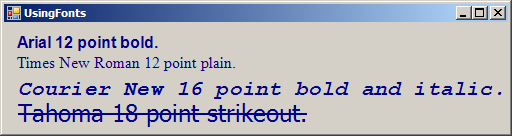
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class UsingFonts : System.Windows.Forms.Form
{
private System.ComponentModel.Container components = null;
public UsingFonts()
{
InitializeComponent();
}
private void InitializeComponent()
{
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(504, 109);
this.Name = "UsingFonts";
this.Text = "UsingFonts";
}
static void Main()
{
Application.Run( new UsingFonts() );
}
protected override void OnPaint(PaintEventArgs paintEvent )
{
Graphics graphicsObject = paintEvent.Graphics;
SolidBrush brush = new SolidBrush( Color.DarkBlue );
// arial, 12 pt bold
FontStyle style = FontStyle.Bold;
Font arial = new Font( new FontFamily( "Arial" ), 12, style );
// times new roman, 12 pt regular
style = FontStyle.Regular;
Font timesNewRoman = new Font( "Times New Roman", 12, style );
// courier new, 16 pt bold and italic
style = FontStyle.Bold | FontStyle.Italic;
Font courierNew = new Font( "Courier New", 16, style );
// tahoma, 18 pt strikeout
style = FontStyle.Strikeout;
Font tahoma = new Font( "Tahoma", 18, style );
graphicsObject.DrawString( arial.Name +
" 12 point bold.", arial, brush, 10, 10 );
graphicsObject.DrawString( timesNewRoman.Name +
" 12 point plain.", timesNewRoman, brush, 10, 30 );
graphicsObject.DrawString( courierNew.Name +
" 16 point bold and italic.", courierNew,
brush, 10, 54 );
graphicsObject.DrawString( tahoma.Name +
" 18 point strikeout.", tahoma, brush, 10, 75 );
}
}
Related examples in the same category