Font Viewer
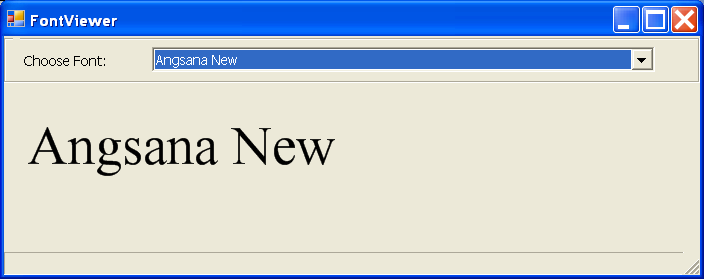
/*
User Interfaces in C#: Windows Forms and Custom Controls
by Matthew MacDonald
Publisher: Apress
ISBN: 1590590457
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using Microsoft.Win32;
namespace FontViewer
{
/// <summary>
/// Summary description for Form1.
/// </summary>
public class FontViewer : System.Windows.Forms.Form
{
private System.Windows.Forms.GroupBox groupBox1;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.ComboBox lstFonts;
private System.Windows.Forms.StatusBar statusBar;
private System.Windows.Forms.StatusBarPanel panel;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public FontViewer()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
// System.Resources.ResourceManager resources = new System.Resources.ResourceManager(typeof(FontViewer));
this.groupBox1 = new System.Windows.Forms.GroupBox();
this.label1 = new System.Windows.Forms.Label();
this.lstFonts = new System.Windows.Forms.ComboBox();
this.statusBar = new System.Windows.Forms.StatusBar();
this.panel = new System.Windows.Forms.StatusBarPanel();
this.groupBox1.SuspendLayout();
((System.ComponentModel.ISupportInitialize)(this.panel)).BeginInit();
this.SuspendLayout();
//
// groupBox1
//
this.groupBox1.Anchor = ((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right);
this.groupBox1.Controls.AddRange(new System.Windows.Forms.Control[] {
this.lstFonts,
this.label1});
this.groupBox1.Location = new System.Drawing.Point(0, -4);
this.groupBox1.Name = "groupBox1";
this.groupBox1.Size = new System.Drawing.Size(632, 40);
this.groupBox1.TabIndex = 0;
this.groupBox1.TabStop = false;
//
// label1
//
this.label1.Location = new System.Drawing.Point(12, 16);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(80, 12);
this.label1.TabIndex = 0;
this.label1.Text = "Choose Font:";
//
// lstFonts
//
this.lstFonts.DropDownStyle = System.Windows.Forms.ComboBoxStyle.DropDownList;
this.lstFonts.DropDownWidth = 340;
this.lstFonts.Location = new System.Drawing.Point(100, 12);
this.lstFonts.Name = "lstFonts";
this.lstFonts.Size = new System.Drawing.Size(340, 21);
this.lstFonts.TabIndex = 1;
this.lstFonts.SelectedIndexChanged += new System.EventHandler(this.lstFonts_SelectedIndexChanged);
//
// statusBar
//
this.statusBar.Location = new System.Drawing.Point(0, 165);
this.statusBar.Name = "statusBar";
this.statusBar.Panels.AddRange(new System.Windows.Forms.StatusBarPanel[] {
this.panel});
this.statusBar.ShowPanels = true;
this.statusBar.Size = new System.Drawing.Size(632, 20);
this.statusBar.TabIndex = 1;
//
// panel
//
this.panel.AutoSize = System.Windows.Forms.StatusBarPanelAutoSize.Spring;
this.panel.Width = 616;
//
// FontViewer
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 14);
this.ClientSize = new System.Drawing.Size(632, 185);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.statusBar,
this.groupBox1});
this.Font = new System.Drawing.Font("Tahoma", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
// this.Icon = ((System.Drawing.Icon)(resources.GetObject("$this.Icon")));
this.Name = "FontViewer";
this.Text = "FontViewer";
this.Load += new System.EventHandler(this.FontViewer_Load);
this.Paint += new System.Windows.Forms.PaintEventHandler(this.FontViewer_Paint);
this.groupBox1.ResumeLayout(false);
((System.ComponentModel.ISupportInitialize)(this.panel)).EndInit();
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// The FontViewer entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new FontViewer());
}
private void FontViewer_Load(object sender, System.EventArgs e)
{
System.Drawing.Text.InstalledFontCollection fonts = new System.Drawing.Text.InstalledFontCollection();
foreach (FontFamily family in fonts.Families)
{
lstFonts.Items.Add(family.Name);
}
RegistryKey rk;
rk = Registry.LocalMachine.OpenSubKey("Software\\ProseTech\\FontViewer");
if (rk != null) this.Text += " - " + rk.GetValue("Customer");
}
private void FontViewer_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
if (lstFonts.Text != "")
{
try
{
e.Graphics.DrawString(lstFonts.Text, new Font(lstFonts.Text, 50), Brushes.Black, 10, 50);
statusBar.Panels[0].Text = "";
}
catch (Exception err)
{
statusBar.Panels[0].Text = err.Message;
}
}
}
private void lstFonts_SelectedIndexChanged(object sender, System.EventArgs e)
{
if (lstFonts.Text != "") this.Invalidate();
}
}
}
Related examples in the same category