Node Relationships:childNodes
All nodes in a document have relationships to other nodes.
For the following html code:
<html>
<head>
<title>Sample Page</title>
</head>
<body>
<p id='myP'>Hello World!</p>
</body>
</html>
Its DOM tree is
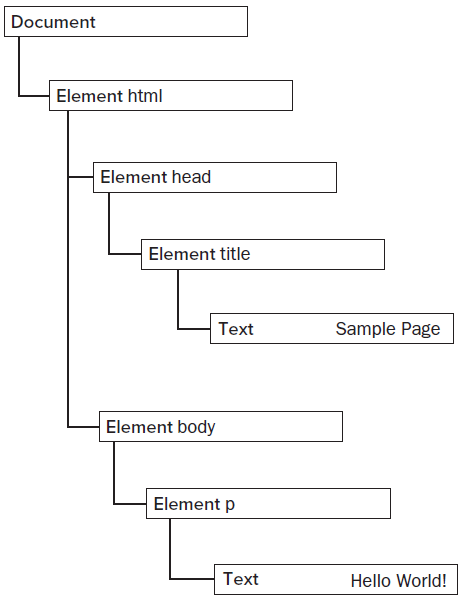
In HTML, the <body> element is considered a child of the <html> element. The <html> element is considered the parent of the <body> element. The <head> element is considered a sibling of the <body> element.
Each node has a childNodes property containing a NodeList. A NodeList is an array-like object used to store an ordered list of nodes that are accessible by position.
The following example shows how nodes stored in a NodeList may be accessed via bracket notation or by using the item() method:
<!DOCTYPE HTML>
<html>
<head>
<title>Example</title>
</head>
<body>
<pre id="results"><code><code></pre>
<script>
var resultsElement = document.getElementById("results");
document.writeln(resultsElement.nodeType);
document.writeln(resultsElement.nodeName);
var firstChild = resultsElement.childNodes[0];
document.writeln(firstChild.nodeName);
</script>
</body>
</html>
By item
<!DOCTYPE HTML>
<html>
<head>
<title>Example</title>
</head>
<body>
<div id="results">
<code><code>
<p></p>
</div>
<script>
var resultsElement = document.getElementById("results");
document.writeln(resultsElement.nodeType);
document.writeln(resultsElement.nodeName);
var secondChild = resultsElement.childNodes.item(1);
document.writeln(secondChild.nodeName);
</script>
</body>
</html>
Home
JavaScript Book
DOM
JavaScript Book
DOM
DOM Model:
- Document Object Model
- nodeName and nodeValue Properties
- Node Relationships:childNodes
- parentNode
- previousSibling and nextSibling properties
- lastChild and firstChild
- appendChild() adds a node to the end of the childNodes list
- insertBefore()
- ownerDocument property
- removeChild
- replaceChild()
- Working with Text
- Check the length of NodeList
- Convert NodeList to an Array
- html tag and its cooresponding JavaScript class