Document Object Model
HTML or XML document can be represented as a hierarchy of nodes using the DOM.
For the following html code:
<html>
<head>
<title>Sample Page</title>
</head>
<body>
<p id='myP'>Hello World!</p>
</body>
</html>
Its DOM tree is
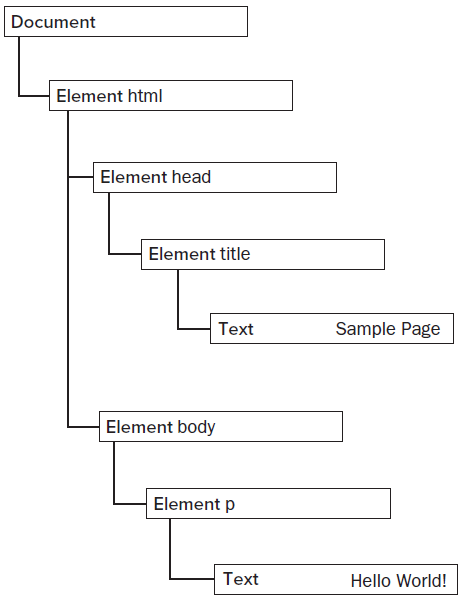
The root is document node. The only child of the document node is the <html> element.
<html> element is called the document element. The document element is the outermost element.
p is an HTML element and body is also an HTML element. myP is an attribute.
HTML elements are represented by element nodes. attributes are represented by attribute nodes
Each node has a type. Nodes have node type, attributes have node type and comments have comment type. The element node and attribute are different in term of type.
In total, there are 12 node types, all of which inherit from a base type.
The Node Type
The Node interface is implemented in JavaScript as the Node type. All node types inherit from Node in JavaScript. All node types share the same basic properties and methods.
Every node has a nodeType property that indicates the type of node that it is. Node types are represented by one of the following 12 numeric constants on the Node type:
Type | Value |
---|---|
Node.ELEMENT_NODE | 1 |
Node.ATTRIBUTE_NODE | 2 |
Node.TEXT_NODE | 3 |
Node.CDATA_SECTION_NODE | 4 |
Node.ENTITY_REFERENCE_NODE | 5 |
Node.ENTITY_NODE | 6 |
Node.PROCESSING_INSTRUCTION_NODE | 7 |
Node.COMMENT_NODE | 8 |
Node.DOCUMENT_NODE | 9 |
Node.DOCUMENT_TYPE_NODE | 10 |
Node.DOCUMENT_FRAGMENT_NODE | 11 |
Node.NOTATION_NODE | 12 |
A node's type is easy to determine by comparing against one of these constants:
<!DOCTYPE HTML>
<html>
<head>
<title>Example</title>
</head>
<body>
<pre id="results"></pre>
<script>
var resultsElement = document.getElementById("results");
document.writeln(resultsElement.nodeType);
document.writeln(resultsElement.nodeName);
if (resultsElement.nodeType == Node.ELEMENT_NODE){ //won't work in IE < 9
document.writeln("Node is an element.");
}
</script>
</body>
</html>
For cross-browser compatibility, it's best to compare the nodeType property against a numeric value:
<!DOCTYPE HTML>
<html>
<head>
<title>Example</title>
</head>
<body>
<pre id="results"></pre>
<script>
var resultsElement = document.getElementById("results");
document.writeln(resultsElement.nodeType);
document.writeln(resultsElement.nodeName);
if (resultsElement.nodeType == 1){
document.writeln("Node is an element.");
}
</script>
</body>
</html>
JavaScript Book
DOM
- Document Object Model
- nodeName and nodeValue Properties
- Node Relationships:childNodes
- parentNode
- previousSibling and nextSibling properties
- lastChild and firstChild
- appendChild() adds a node to the end of the childNodes list
- insertBefore()
- ownerDocument property
- removeChild
- replaceChild()
- Working with Text
- Check the length of NodeList
- Convert NodeList to an Array
- html tag and its cooresponding JavaScript class