Add fragment
Description
The following code shows you the basic usage of fragments.
Example
In the res/layout
folder, add a new file and name it fragment1.xml
.
Populate it with the following:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout/*from ww w .ja v a 2 s. c o m*/
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#00FF00"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="This is fragment #1"
android:textColor="#000000"
android:textSize="25sp" />
</LinearLayout>
Also in the res/layout
folder, add another new file and name it fragment2.xml
.
Populate it as follows:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout//from w w w .j a va2 s. com
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#FFFE00"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="This is fragment #2"
android:textColor="#000000"
android:textSize="25sp" />
</LinearLayout>
In activity_main.xml
, add the following code:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="horizontal" >
/*from w w w . j av a 2 s . c o m*/
<fragment
android:name="com.java2s.myapplication3.app.Fragment1"
android:id="@+id/fragment1"
android:layout_weight="1"
android:layout_width="0px"
android:layout_height="match_parent" />
<fragment
android:name="com.java2s.myapplication3.app.Fragment2"
android:id="@+id/fragment2"
android:layout_weight="1"
android:layout_width="0px"
android:layout_height="match_parent" />
</LinearLayout>
Under the com.java2s.Fragments
package name, add two
Java class files and name them Fragment1.java
and Fragment2.java
Add the following code to Fragment1.java
:
package com.java2s.myapplication3.app;
//w w w .ja va2 s. c om
import android.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class Fragment1 extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater,
ViewGroup container, Bundle savedInstanceState) {
//Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment1, container, false);
}
}
Add the following code to Fragment2.java
:
package com.java2s.myapplication3.app;
/*from w w w . j av a2 s .co m*/
import android.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class Fragment2 extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater,
ViewGroup container, Bundle savedInstanceState) {
//Inflate the layout for this fragment
return inflater.inflate(
R.layout.fragment2, container, false);
}
}
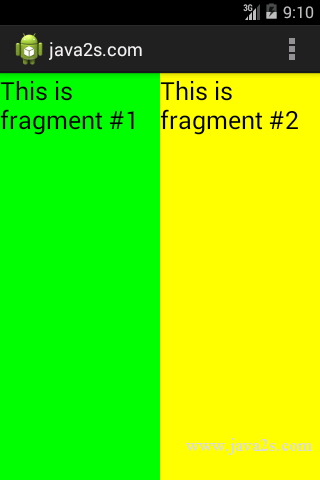
Note
A fragment behaves very much like an activity: it has
- a Java class
- it loads its UI from an XML file.
The XML file contains all the usual UI elements that you expect from an activity: TextView, EditText, Button, and so on.
The Java class for a fragment needs to extend the Fragment
base class:
public class Fragment1 extends Fragment {
}
Besides the Fragment
base class, a fragment can also extend a few other
subclasses of the Fragment
class, such as DialogFragment
,
ListFragment
, and
PreferenceFragment
.
To draw the UI for a fragment, you override the onCreateView()
method.
This method needs to return a View object.
You can use a LayoutInflater object to inflate the UI from the specified XML file.
To add a fragment to an activity, you use the <fragment> element:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="horizontal" >
/*from w w w . ja va2 s .c om*/
<fragment
android:name="com.java2s.Fragments.Fragment1"
android:id="@+id/fragment1"
android:layout_weight="1"
android:layout_width="0px"
android:layout_height="match_parent" />
<fragment
android:name="com.java2s.Fragments.Fragment2"
android:id="@+id/fragment2"
android:layout_weight="1"
android:layout_width="0px"
android:layout_height="match_parent" />
</LinearLayout>
Each fragment needs a unique identifier.