CheckBox state change event
Description
To implement specific logic when a check box is checked or unchecked, you
can register for the on-checked event by calling
setOnCheckedChangeListener()
with an
implementation of the OnCheckedChangeListener
interface.
Then implement
the onCheckedChanged()
method, which will
be called when the check box is checked or
unchecked.
Example
Java code
package com.java2s.app;
/* www. j ava2s. co m*/
import android.app.Activity;
import android.os.Bundle;
import android.util.Log;
import android.widget.CheckBox;
import android.widget.CompoundButton;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
CheckBox fishCB = (CheckBox) findViewById(R.id.fishCB);
if (fishCB.isChecked()) {
fishCB.toggle(); // flips the checkbox to unchecked if it was checked
}
fishCB.setOnCheckedChangeListener(
new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton arg0, boolean isChecked) {
Log.v("CheckBoxActivity", "The fish checkbox is now "
+ (isChecked ? "checked" : "not checked"));
}
}
);
}
}
XML layout file.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical">
//from www .jav a 2 s .c o m
<CheckBox
android:id="@+id/chickenCB"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:checked="true"
android:text="XML" />
<CheckBox
android:id="@+id/fishCB"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="CSS" />
<CheckBox
android:id="@+id/steakCB"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:checked="true"
android:text="HTML" />
</LinearLayout>
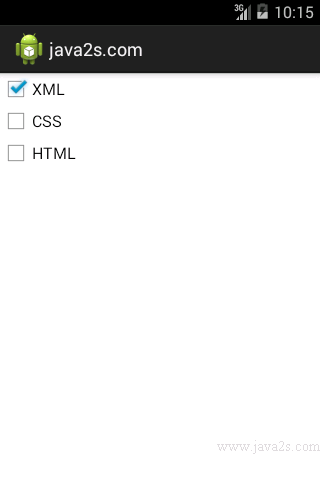