<Window x:Class="Commands.SimpleDocument"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="SimpleDocument" Height="300" Width="300">
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto"></RowDefinition>
<RowDefinition Height="Auto"></RowDefinition>
<RowDefinition></RowDefinition>
</Grid.RowDefinitions>
<Menu Grid.Row="0">
<MenuItem Header="File">
<MenuItem Command="New"></MenuItem>
<MenuItem Command="Open"></MenuItem>
<MenuItem Command="Save"></MenuItem>
<MenuItem Command="SaveAs"></MenuItem>
<Separator></Separator>
<MenuItem Command="Close"></MenuItem>
</MenuItem>
</Menu>
<ToolBarTray Grid.Row="1">
<ToolBar>
<Button Command="New">New</Button>
<Button Command="Open">Open</Button>
<Button Command="Save">Save</Button>
</ToolBar>
<ToolBar>
<Button Command="Cut">Cut</Button>
<Button Command="Copy">Copy</Button>
<Button Command="Paste">Paste</Button>
</ToolBar>
</ToolBarTray>
<TextBox Name="txt" Margin="5" Grid.Row="2"
TextWrapping="Wrap" AcceptsReturn="True"
TextChanged="txt_TextChanged"></TextBox>
</Grid>
</Window>
//File:Window.xaml.vb
Imports System
Imports System.Collections.Generic
Imports System.Text
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Data
Imports System.Windows.Documents
Imports System.Windows.Input
Imports System.Windows.Media
Imports System.Windows.Media.Imaging
Imports System.Windows.Shapes
Namespace Commands
Public Partial Class SimpleDocument
Inherits System.Windows.Window
Public Sub New()
InitializeComponent()
Dim binding As CommandBinding
binding = New CommandBinding(ApplicationCommands.[New])
AddHandler binding.Executed, AddressOf NewCommand
Me.CommandBindings.Add(binding)
binding = New CommandBinding(ApplicationCommands.Open)
AddHandler binding.Executed, AddressOf OpenCommand
Me.CommandBindings.Add(binding)
binding = New CommandBinding(ApplicationCommands.Save)
AddHandler binding.Executed, AddressOf SaveCommand_Executed
AddHandler binding.CanExecute, AddressOf SaveCommand_CanExecute
Me.CommandBindings.Add(binding)
End Sub
Private Sub NewCommand(sender As Object, e As ExecutedRoutedEventArgs)
Console.WriteLine("New command triggered with " & e.Source.ToString())
isDirty = False
End Sub
Private Sub OpenCommand(sender As Object, e As ExecutedRoutedEventArgs)
isDirty = False
End Sub
Private Sub SaveCommand_Executed(sender As Object, e As ExecutedRoutedEventArgs)
Console.WriteLine("Save command triggered with " & e.Source.ToString())
isDirty = False
End Sub
Private isDirty As Boolean = False
Private Sub txt_TextChanged(sender As Object, e As RoutedEventArgs)
isDirty = True
End Sub
Private Sub SaveCommand_CanExecute(sender As Object, e As CanExecuteRoutedEventArgs)
e.CanExecute = isDirty
End Sub
End Class
End Namespace
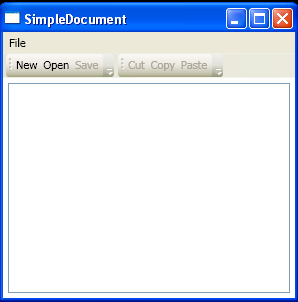