<Window x:Class="WpfApplication1.PerFrameAnimation"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Per Frame Animation" Height="400" Width="400">
<Canvas>
<Path Fill="Blue">
<Path.Data>
<EllipseGeometry x:Name="ball1" Center="30,180" RadiusX="5" RadiusY="5" />
</Path.Data>
</Path>
<Path Stroke="LightBlue">
<Path.Data>
<EllipseGeometry Center="180,180" RadiusX="150" RadiusY="75" />
</Path.Data>
</Path>
</Canvas>
</Window>
//File:Window.xaml.vb
Imports System
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Media
Imports System.Windows.Media.Animation
Imports System.Windows.Shapes
Namespace WpfApplication1
Public Partial Class PerFrameAnimation
Inherits Window
Private lastRender As TimeSpan
Private time As Double = 0
Private dt As Double = 0
Public Sub New()
lastRender = TimeSpan.FromTicks(DateTime.Now.Ticks)
AddHandler CompositionTarget.Rendering, AddressOf StartAnimation
End Sub
Private Sub StartAnimation(sender As Object, e As EventArgs)
Dim renderArgs As RenderingEventArgs = DirectCast(e, RenderingEventArgs)
dt = (renderArgs.RenderingTime - lastRender).TotalSeconds
lastRender = renderArgs.RenderingTime
Dim x As Double = 180 + 150 * Math.Cos(2 * time)
Dim y As Double = 180 + 75 * Math.Sin(2 * time)
ball1.Center = New Point(x, y)
time += dt
End Sub
End Class
End Namespace
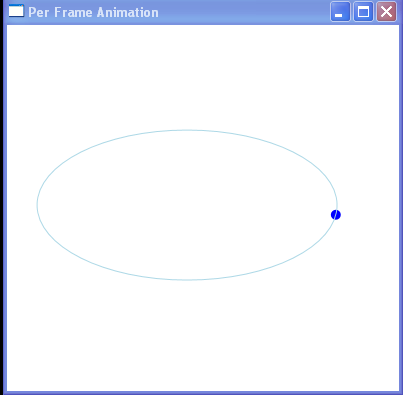