<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="WPF" Height="300" Width="300">
<StackPanel>
<TextBox AcceptsReturn="True" Height="100" IsReadOnly="True"
Name="textBox1" TextAlignment="Left" TextWrapping="Wrap"
VerticalScrollBarVisibility="Auto">
Default starting text.
</TextBox>
<WrapPanel Margin="10">
<Button Margin="5" Name="textButton" Width="75" Click="TextButton_Click">Set Text</Button>
<Button Margin="5" Name="selectAllButton" Width="75" Click="SelectAllButton_Click">Select All</Button>
<Button Margin="5" Name="clearButton" Width="75" Click="ClearButton_Click">Clear</Button>
<Button Margin="5" Name="prependButton" Width="75" Click="PrependButton_Click">Prepend</Button>
<Button Margin="5" Name="insertButton" Width="75" Click="InsertButton_Click">Insert</Button>
<Button Margin="5" Name="appendButton" Width="75" Click="AppendButton_Click">Append</Button>
<Button Margin="5" Name="cutButton" Width="75" Click="CutButton_Click">Cut</Button>
<Button Margin="5" Name="pasteButton" Width="75" Click="PasteButton_Click">Paste</Button>
<Button Margin="5" Name="undoButton" Width="75" Click="UndoButton_Click">Undo</Button>
</WrapPanel>
</StackPanel>
</Window>
//File:Window.xaml.vb
Imports System.Windows
Imports System.Windows.Controls
Namespace WpfApplication1
Public Partial Class Window1
Inherits Window
Public Sub New()
InitializeComponent()
End Sub
Private Sub AppendButton_Click(sender As Object, e As RoutedEventArgs)
textBox1.AppendText("text")
End Sub
Private Sub ClearButton_Click(sender As Object, e As RoutedEventArgs)
textBox1.Clear()
End Sub
Private Sub CutButton_Click(sender As Object, e As RoutedEventArgs)
If textBox1.SelectionLength = 0 Then
MessageBox.Show("Select text to cut first.", Title)
Else
MessageBox.Show("Cut: " + textBox1.SelectedText, Title)
textBox1.Cut()
End If
End Sub
Private Sub InsertButton_Click(sender As Object, e As RoutedEventArgs)
textBox1.Text = textBox1.Text.Insert(textBox1.CaretIndex, "text")
End Sub
Private Sub PasteButton_Click(sender As Object, e As RoutedEventArgs)
textBox1.Paste()
End Sub
Private Sub PrependButton_Click(sender As Object, e As RoutedEventArgs)
textBox1.Text = textBox1.Text.Insert(0, "Prepend")
End Sub
Private Sub SelectAllButton_Click(sender As Object, e As RoutedEventArgs)
textBox1.SelectAll()
textBox1.Focus()
End Sub
Private Sub TextButton_Click(sender As Object, e As RoutedEventArgs)
textBox1.Text = "new value"
End Sub
Private Sub UndoButton_Click(sender As Object, e As RoutedEventArgs)
textBox1.Undo()
End Sub
End Class
End Namespace
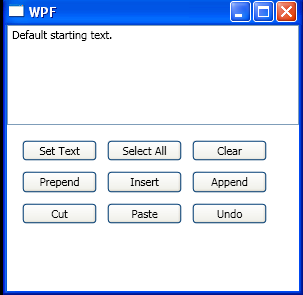
16.4.TextBox |
| 16.4.1. | You cannot use TextBox and Image at the same time for Button Content |  |
| 16.4.2. | Single line and Multiline TextBox | 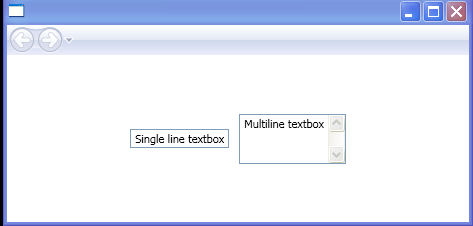 |
| 16.4.3. | TextBox Column | 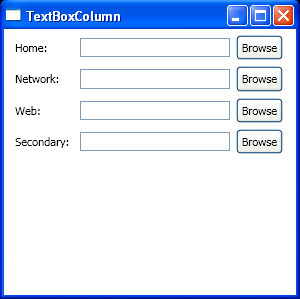 |
| 16.4.4. | Bind value to TextBox | 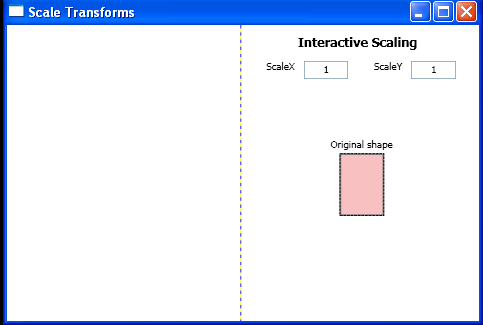 |
| 16.4.5. | An upside down TextBox | 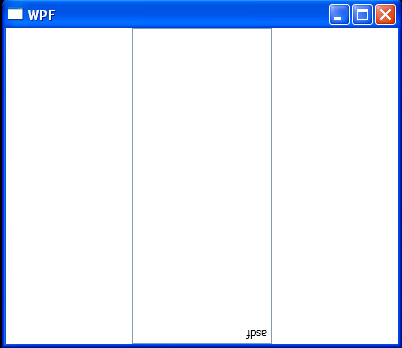 |
| 16.4.6. | Style with Data Trigger for TextBox | 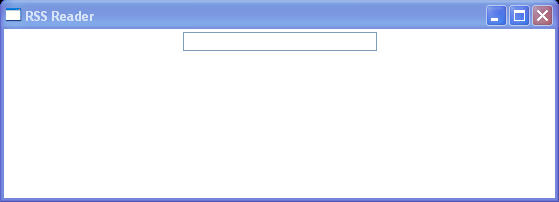 |
| 16.4.7. | TextBox with custom ErrorTemplate and ToolTip | 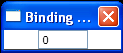 |
| 16.4.8. | TextBox uses the ExceptionValidationRule and UpdateSourceExceptionFilter handler | 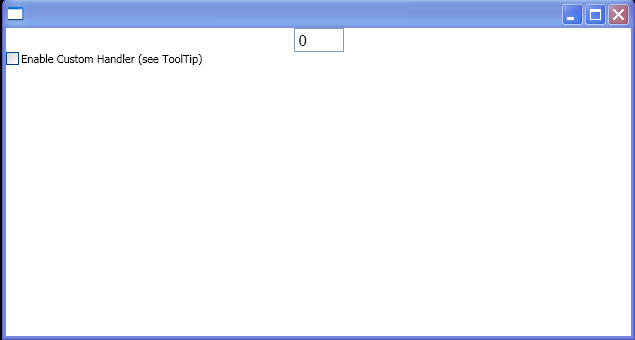 |
| 16.4.9. | TextBox with UpdateSourceExceptionFilter handler | 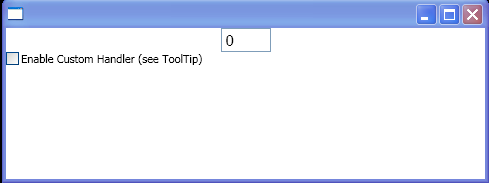 |
| 16.4.10. | TextBox focus listener | 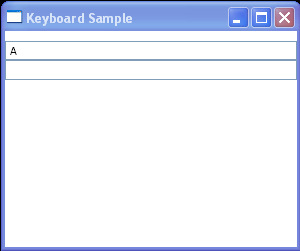 |
| 16.4.11. | TextBox MouseLeftButtonDown action and PreviewMouseLeftButtonDown action | 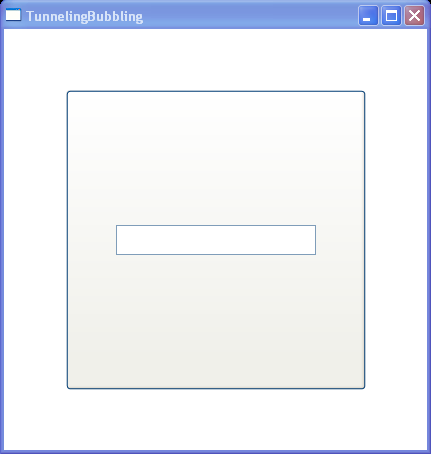 |
| 16.4.12. | Mark the text control as being changed to prevent any text content or selection changed events | 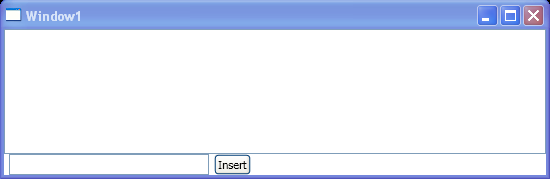 |
| 16.4.13. | Listen to TextBox text changed event | 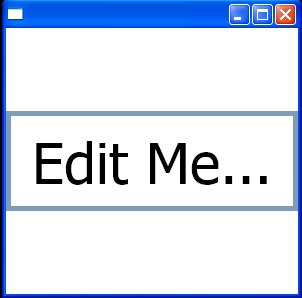 |
| 16.4.14. | Handler for the PreviewKeyDown event on the TextBox | 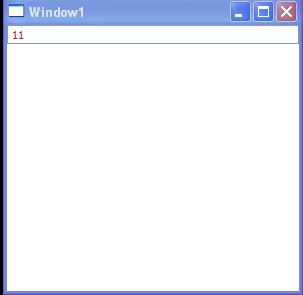 |
| 16.4.15. | Format TextBox with MenuItem: normal, bold, italic | 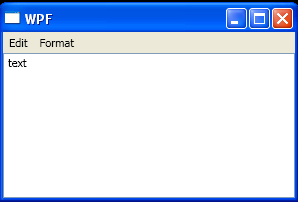 |
| 16.4.16. | TextBox: set text, select all, clear, prepend, insert, append, cut, paste, undo | 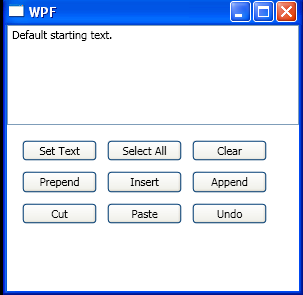 |
| 16.4.17. | Set TextBox to editable | 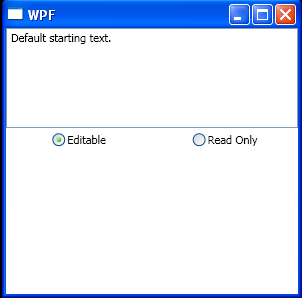 |
| 16.4.18. | Scroll TextBox |  |
| 16.4.19. | Use TextBox.CommandBindingst to bind command | 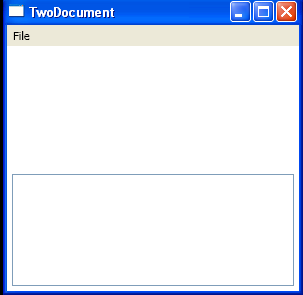 |
| 16.4.20. | Use Dictionary to record which textbox has been changed and not saved | 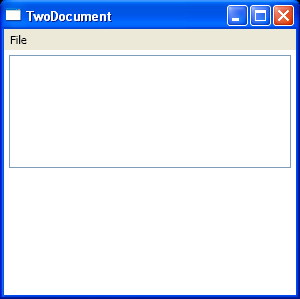 |
| 16.4.21. | Set TextBox ContextMenu to null | 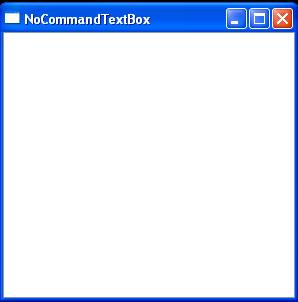 |
| 16.4.22. | TextBox Selection start, end and selected text | 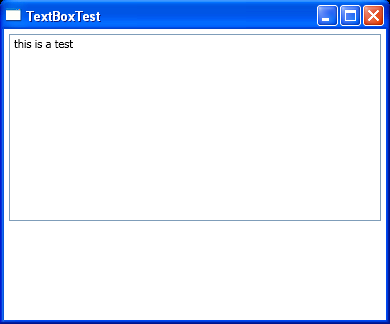 |
| 16.4.23. | Scrollable TextBox Column | 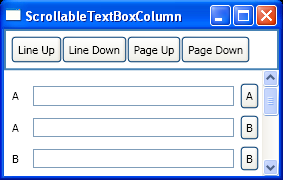 |
| 16.4.24. | Check Spelling Error | 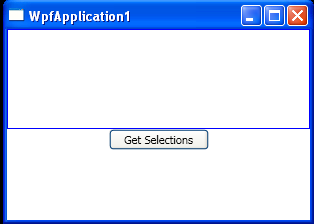 |