<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="WPF" Height="300" Width="300">
<StackPanel>
<ListBox FontSize="16" Height="150" Margin="5" Name="listBox1" SelectionMode="Extended">
<ListBoxItem>List Item 1</ListBoxItem>
<ListBoxItem>List Item 2</ListBoxItem>
<ListBoxItem>List Item 3</ListBoxItem>
</ListBox>
<StackPanel HorizontalAlignment="Center" Orientation="Horizontal">
<Label Content="_New item text:" VerticalAlignment="Center" Target="{Binding ElementName=textBox}" />
<TextBox Margin="5" Name="textBox" MinWidth="120" />
</StackPanel>
<StackPanel HorizontalAlignment="Center" Orientation="Horizontal">
<Button Click="btnAddListItem_Click" Content="Add Item" IsDefault="True" Margin="5" Name="btnAddListItem" />
<Button Click="btnDeleteListItem_Click" Content="Delete Items" Margin="5" Name="btnDeleteListItem" />
<Button Click="btnSelectAll_Click" Content="Select All" Margin="5" Name="btnSelectAll" />
</StackPanel>
</StackPanel>
</Window>
//File:Window.xaml.vb
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Media
Namespace WpfApplication1
Public Partial Class Window1
Inherits Window
Public Sub New()
InitializeComponent()
End Sub
Private Sub btnAddListItem_Click(sender As Object, e As RoutedEventArgs)
If textBox.Text.Length = 0 Then
MessageBox.Show("Enter text to add to the list.", Title)
Else
Dim item As New ListBoxItem()
item.Content = textBox.Text
item.IsSelected = True
item.HorizontalAlignment = HorizontalAlignment.Center
item.FontWeight = FontWeights.Bold
item.FontFamily = New FontFamily("Tahoma")
listBox1.Items.Add(item)
textBox.Clear()
textBox.Focus()
End If
End Sub
Private Sub btnDeleteListItem_Click(sender As Object, e As RoutedEventArgs)
If listBox1.SelectedItems.Count = 0 Then
MessageBox.Show("Select list items to delete.", Title)
Else
While listBox1.SelectedItems.Count > 0
listBox1.Items.Remove(listBox1.SelectedItems(0))
End While
End If
End Sub
Private Sub btnSelectAll_Click(sender As Object, e As RoutedEventArgs)
listBox1.SelectAll()
End Sub
End Class
End Namespace
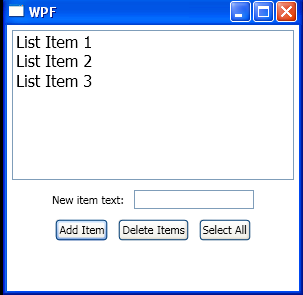